You need to implement a class for Dequeue i.e. for double ended queue. In this queue, elements can be inserted and deleted from both the ends. You don't need to double the capacity. You need to implement the following functions - 1. constructor You need to create the appropriate constructor. Size for the queue passed is 10. 2. insertFront - This function takes an element as input and insert the element at the front of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. 3. insertRear - This function takes an element as input and insert the element at the end of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. 4. deleteFront - This function removes an element from the front of queue. Print -1 if queue is empty. 5. deleteRear - This function removes an element from the end of queue. Print -1 if queue is empty. 6. getFront - Returns the element which is at front of the queue. Return -1 if queue is empty. 7. getRear - Returns the element which is at end of the queue. Return -1 if queue is empty. Input Format: For C++ and Java, input is already managed for you. You just have to implement given functions. For Python: The choice codes and corresponding input for each choice are given in a new line. The input is terminated by integer -1. The following table elaborately describes the function, their choice codes and their corresponding input - Alt Text Output Format: For C++ and Java, output is already managed for you. You just have to implement given functions. For Python: The output format for each function has been mentioned in the problem description itself. Sample Input 1: 5 1 49 1 64 2 99 5 6 -1 Sample Output 1: -1 64 99 Explanation: The first choice code corresponds to getFront. Since the queue is empty, hence the output is -1. The following input adds 49 at the top and the resultant queue becomes: 49. The following input adds 64 at the top and the resultant queue becomes: 64 -> 49 The following input add 99 at the end and the resultant queue becomes: 64 -> 49 -> 99 The following input corresponds to getFront. Hence the output is 64. The following input corresponds to getRear. Hence the output is 99..
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
You need to implement a class for Dequeue i.e. for double ended queue. In this queue, elements can be inserted and deleted from both the ends. | |
You don't need to double the capacity. | |
You need to implement the following functions - | |
1. constructor | |
You need to create the appropriate constructor. Size for the queue passed is 10. | |
2. insertFront - | |
This function takes an element as input and insert the element at the front of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. | |
3. insertRear - | |
This function takes an element as input and insert the element at the end of queue. Insert the element only if queue is not full. And if queue is full, print -1 and return. | |
4. deleteFront - | |
This function removes an element from the front of queue. Print -1 if queue is empty. | |
5. deleteRear - | |
This function removes an element from the end of queue. Print -1 if queue is empty. | |
6. getFront - | |
Returns the element which is at front of the queue. Return -1 if queue is empty. | |
7. getRear - | |
Returns the element which is at end of the queue. Return -1 if queue is empty. | |
Input Format: | |
For C++ and Java, input is already managed for you. You just have to implement given functions. | |
For Python: | |
The choice codes and corresponding input for each choice are given in a new line. The input is terminated by integer -1. The following table elaborately describes the function, their choice codes and their corresponding input - | |
Alt Text | |
Output Format: | |
For C++ and Java, output is already managed for you. You just have to implement given functions. | |
For Python: | |
The output format for each function has been mentioned in the problem description itself. | |
Sample Input 1: | |
5 1 49 1 64 2 99 5 6 -1 | |
Sample Output 1: | |
-1 | |
64 | |
99 | |
Explanation: | |
The first choice code corresponds to getFront. Since the queue is empty, hence the output is -1. | |
The following input adds 49 at the top and the resultant queue becomes: 49. | |
The following input adds 64 at the top and the resultant queue becomes: 64 -> 49 | |
The following input add 99 at the end and the resultant queue becomes: 64 -> 49 -> 99 | |
The following input corresponds to getFront. Hence the output is 64. | |
The following input corresponds to getRear. Hence the output is 99..
|

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

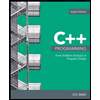
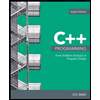