TO DO: Code connection servers for all phones code: """ HYPHEN = "-" QUIT = 'quit' SWITCH_CONNECT = 'switch-connect' SWITCH_ADD = 'switch-add' PHONE_ADD = 'phone-add' NETWORK_SAVE = 'network-save' NETWORK_LOAD = 'network-load' START_CALL = 'start-call' END_CALL = 'end-call' DISPLAY = 'display' def connect_switchboards(switchboards, area_1, area_2): pass def add_switchboard(switchboards, area_code): pass def add_phone(switchboards, area_code, phone_number): pass def save_network(switchboards, file_name): pass def load_network(file_name): """ :param file_name: the name of the file to load. :return: you must return the new switchboard network. If you don't, then it won't load properly. """ pass def start_call(switchboards, start_area, start_number, end_area, end_number): pass def end_call(switchboards, start_area, start_number): pass def display(switchboards): pass FUNCTIONS: switch-add [area-code-1] This will create a new switchboard with the area code specified. The braces are just to indicate that it needs to be replaced with an area code which is a number. If the switchboard exists, you don't create a new one. switch-connect [area-code-1] [area-code-2] This connects the switchboard at [area-code-1] with the one at [area-code-2], and also the reverse connection, which means that they are connected to each other. Calls can flow in both directions. phone-add phone-add [area-code-1]-[num_part_1]-[num_part_2] phone-add should add a phone number with the area code specified as well as the phone number with one hyphen between the area code and the phone number, but there can be as many hyphens between any other part of the number. The rest of the number can be combined, or kept as a format with hyphens. start-call start-call [phone_number_1] [phone_number_2] Connecting a call requires a recursive function. It can be a helper function rather than start_call() start-call should create a connection between the two phone numbers, if the area codes exist, the numbers exist at their proper area codes, and if they are not already in a phone call. It can only be connected if there is a path along switchboards between the two numbers. It is possible that no connection will exist because the two switchboards could not be connected by "trunk lines." end-call end-call [phone_number] end-call should disconnect the two numbers that are connected. The disconnection can occur at either end of the call. If there's no connection from that number, then report that but you don't need to do anything else. display The display command should output each switchboard with its area-code. Then output the other area codes/switchboards that it's connected to. Finally output each local phone number and if it is on the hook, or if it's connected to another phone. See the sample output for more on how I think it should generally look to make it readable for the console output. network-save [filename] This should save the network in a file. CAN USE CSV OR JSON FILE. You must decide on the file format, which must be able to be saved, and then loaded in a different run of the program. The structure of the network should be saved, but the phone to phone connections can be lost (pretend that all phones are hung up at the start of the load). network-load [filename] This should load the file at file-name. When you load a network, you should delete the old network or overwrite it, whichever makes sense for your project. Output Enter command: switch-add 443 Enter command: switch-add 410 Enter command: switch-add 656 Enter command: display Switchboard with area code: 443 Trunk lines are: Local phone numbers are: Switchboard with area code: 410 Trunk lines are: Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Local phone numbers are: Enter command: switch-connect 443 410 Enter command: phone-add 656-112-3412 Enter command: phone-add 443-132-1332 Enter command: display Switchboard with area code: 443 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1321332 is not in use. Switchboard with area code: 410 Trunk lines are: Trunk line connection to: 443 Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Local phone numbers are: Phone with number: 1123412 is not in use. Enter command: start-call 656-112-3412 443-132-1332 656-112-3412 and 443-132-1332 were not connected. Enter command: switch-connect 656 410 Enter command: start-call 656-112-3412 443-132-1332 656-112-3412 and 443-132-1332 are now connected. Enter command: display Switchboard with area code: 443 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1321332 is connected to 656-1123412 Switchboard with area code: 410 Trunk lines are: Trunk line connection to: 443 Trunk line connection to: 656 Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1123412 is connected to 443-1321332 Enter command: network-save sample1.net Network saved to sample1.net. Enter command: quit
TO DO: Code connection servers for all phones
code:
"""
HYPHEN = "-"
QUIT = 'quit'
SWITCH_CONNECT = 'switch-connect'
SWITCH_ADD = 'switch-add'
PHONE_ADD = 'phone-add'
NETWORK_SAVE = 'network-save'
NETWORK_LOAD = 'network-load'
START_CALL = 'start-call'
END_CALL = 'end-call'
DISPLAY = 'display'
def connect_switchboards(switchboards, area_1, area_2):
pass
def add_switchboard(switchboards, area_code):
pass
def add_phone(switchboards, area_code, phone_number):
pass
def save_network(switchboards, file_name):
pass
def load_network(file_name):
"""
:param file_name: the name of the file to load.
:return: you must return the new switchboard network. If you don't, then it won't load properly.
"""
pass
def start_call(switchboards, start_area, start_number, end_area, end_number):
pass
def end_call(switchboards, start_area, start_number):
pass
def display(switchboards):
pass
FUNCTIONS:
switch-add [area-code-1]
This will create a new switchboard with the area code specified. The braces are just to indicate that it needs to be replaced with an area code which is a number. If the switchboard exists, you don't create a new one.
switch-connect [area-code-1] [area-code-2]
This connects the switchboard at [area-code-1] with the one at [area-code-2], and also the reverse connection, which means that they are connected to each other. Calls can flow in both directions.
phone-add
phone-add [area-code-1]-[num_part_1]-[num_part_2]
phone-add should add a phone number with the area code specified as well as the phone number with one hyphen between the area code and the phone number, but there can be as many hyphens between any other part of the number. The rest of the number can be combined, or kept as a format with hyphens.
start-call
start-call [phone_number_1] [phone_number_2]
Connecting a call requires a recursive function. It can be a helper function rather than start_call()
start-call should create a connection between the two phone numbers, if the area codes exist, the numbers exist at their proper area codes, and if they are not already in a phone call. It can only be connected if there is a path along switchboards between the two numbers. It is possible that no connection will exist because the two switchboards could not be connected by "trunk lines."
end-call
end-call [phone_number]
end-call should disconnect the two numbers that are connected. The disconnection can occur at either end of the call. If there's no connection from that number, then report that but you don't need to do anything else.
display
The display command should output each switchboard with its area-code. Then output the other area codes/switchboards that it's connected to. Finally output each local phone number and if it is on the hook, or if it's connected to another phone. See the sample output for more on how I think it should generally look to make it readable for the console output.
network-save [filename]
This should save the network in a file. CAN USE CSV OR JSON FILE. You must decide on the file format, which must be able to be saved, and then loaded in a different run of the program. The structure of the network should be saved, but the phone to phone connections can be lost (pretend that all phones are hung up at the start of the load).
network-load [filename]
This should load the file at file-name. When you load a network, you should delete the old network or overwrite it, whichever makes sense for your project.
Output
Enter command: switch-add 443 Enter command: switch-add 410 Enter command: switch-add 656 Enter command: display Switchboard with area code: 443 Trunk lines are: Local phone numbers are: Switchboard with area code: 410 Trunk lines are: Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Local phone numbers are: Enter command: switch-connect 443 410 Enter command: phone-add 656-112-3412 Enter command: phone-add 443-132-1332 Enter command: display Switchboard with area code: 443 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1321332 is not in use. Switchboard with area code: 410 Trunk lines are: Trunk line connection to: 443 Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Local phone numbers are: Phone with number: 1123412 is not in use. Enter command: start-call 656-112-3412 443-132-1332 656-112-3412 and 443-132-1332 were not connected. Enter command: switch-connect 656 410 Enter command: start-call 656-112-3412 443-132-1332 656-112-3412 and 443-132-1332 are now connected. Enter command: display Switchboard with area code: 443 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1321332 is connected to 656-1123412 Switchboard with area code: 410 Trunk lines are: Trunk line connection to: 443 Trunk line connection to: 656 Local phone numbers are: Switchboard with area code: 656 Trunk lines are: Trunk line connection to: 410 Local phone numbers are: Phone with number: 1123412 is connected to 443-1321332 Enter command: network-save sample1.net Network saved to sample1.net. Enter command: quit |

Step by step
Solved in 4 steps with 5 images

You used forbidden methods. See below. Please update without any of the following in the code.
Forbidden Built-ins/Methods/etc
- break, continue
- methods outside those permitted within allowed types
- for instance str.endswith
- list.index, list.count, etc.
- Keywords you definitely don't need: await, as, assert, async, class, except, finally, global, lambda, nonlocal, raise, try, yield
- The is keyword is forbidden, not because it's necessarily bad, but because it doesn't behave as you might expect (it's not the same as ==).
- built in functions: any, all, breakpoint, callable, classmethod, compile, exec, delattr, divmod, enumerate, filter, map, max, min, isinstance, issubclass, iter, locals, oct, next, memoryview, property, repr, reversed, round, set, setattr, sorted, staticmethod, sum, super, type, vars, zip
- If you have read this section, then you know the secret word is: argumentative.
- exit() or quit()
- If something is not on the allowed list, not on this list, then it is probably forbidden.
- The forbidden list can always be overridden by a particular problem, so if a problem allows something on this list, then it is allowed for that problem.
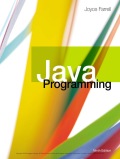
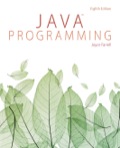
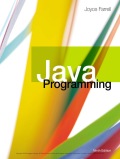
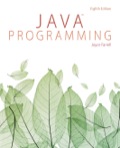