My code from milestone 1 # Define the quiz_type dictionary quiz_type = { 1: "BabyAnimals", 2: "Brooklyn99", 3: "Disney", 4: "Hogwarts", 5: "MyersBriggs", 6: "SesameStreet", 7: "StarWars", 8: "Vegetables" } # Print the welcome message print("Welcome to the Personality Quiz!") print() print("What type of Personality Quiz do you want to run?") print() for number, quiz_name in quiz_type.items(): print(f"{number} - {quiz_name}") print() test_number = int(input("Choose test number (1-8): ")) # Check if the test_number is valid if test_number in quiz_type: quiz_name = quiz_type[test_number] print() print(f"Great! Let's begin the {quiz_name} Personality Quiz...") else: print("Invalid test number. Please choose a number between 1 and 8.") ------------------------------------------------------------------- Milestone #3 code : # Ending statements with the period to easily split them as mentioned in the question. questions = [ "I am the life of the party. E:1", "I warm up quickly to others. A:1 E:1", "I never at a loss for words. O:1 E:1", "I seldom get lost in thought. C:1 O:-1", "I am easily distracted. N:-1 C:-1" ] # Store the printed statement and answer options in string variables welcome_message = "Welcome to the Personality Quiz!" answer_prompt = "Enter your answer here (1-5): " # Function to extract the statement from a question string def extract_statement(question): return question.split('.', 1)[0] # Initialize an empty list to store user answers user_answers = [] # Print the welcome message print(welcome_message + "\n") # Ask the user all five questions and record their answers for i in range(5): # Get the selected question selected_question = questions[i] # Extract the statement from the selected question question_statement = extract_statement(selected_question) # Print the statement with quotation marks and formatting print(f'How much do you agree with this statement?\n"{question_statement}"\n') # Print answer options print("1. Strongly disagree") print("2. Disagree") print("3. Neutral") print("4. Agree") print("5. Strongly agree\n") # Get the user's answer user_answer = int(input(answer_prompt)) # Validate the user input while user_answer < 1 or user_answer > 5: print("Invalid input. Please enter a number between 1 and 5.") user_answer = int(input(answer_prompt)) # Append the user's answer to the list user_answers.append(user_answer) # Print the user's answers print("\nYour answers are:", user_answers) ------------------------------------------------------------ Given code for THIS Milestone to complete with # TODO: Write your header comment here. import driver # Do not remove this quiz_type = {1:"BabyAnimals", 2:"Brooklyn99", 3:"Disney", 4:"Hogwarts", 5:"MyersBriggs", 6:"SesameStreet", 7:"StarWars", 8:"Vegetables"} questions = ["I am the life of the party. E:1", "I warm up quickly to others. A:1 E:1", "I never at a loss for words. O:1 E:1", "I seldom get lost in thought. C:1 O:-1", "I am easily distracted. N:-1 C:-1"] # COPY AND PASTE YOUR CODE FROM MILESTONE #1 AND MILESTONE #3 HERE # TODO: Write your code here. -------------------------------------------------------------------- Follow all directions and the output code should look EXACTLY like this Welcome to the Personality Quiz! ⏎ What type of Personality Quiz do you want to run? ⏎ 1 - BabyAnimals 2 - Brooklyn99 3 - Disney 4 - Hogwarts 5 - MyersBriggs 6 - SesameStreet 7 - StarWars 8 - Vegetables ⏎ Choose test number (1-8): Great! Let's begin the BabyAnimals Personality Quiz... ⏎ How much do you agree with this statement? "I am the life of the party" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I warm up quickly to others" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I never at a loss for words" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I seldom get lost in thought" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I am easily distracted" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): You got Baby Hamster! ⏎ Goodbye.
My code from milestone 1 # Define the quiz_type dictionary quiz_type = { 1: "BabyAnimals", 2: "Brooklyn99", 3: "Disney", 4: "Hogwarts", 5: "MyersBriggs", 6: "SesameStreet", 7: "StarWars", 8: "Vegetables" } # Print the welcome message print("Welcome to the Personality Quiz!") print() print("What type of Personality Quiz do you want to run?") print() for number, quiz_name in quiz_type.items(): print(f"{number} - {quiz_name}") print() test_number = int(input("Choose test number (1-8): ")) # Check if the test_number is valid if test_number in quiz_type: quiz_name = quiz_type[test_number] print() print(f"Great! Let's begin the {quiz_name} Personality Quiz...") else: print("Invalid test number. Please choose a number between 1 and 8.") ------------------------------------------------------------------- Milestone #3 code : # Ending statements with the period to easily split them as mentioned in the question. questions = [ "I am the life of the party. E:1", "I warm up quickly to others. A:1 E:1", "I never at a loss for words. O:1 E:1", "I seldom get lost in thought. C:1 O:-1", "I am easily distracted. N:-1 C:-1" ] # Store the printed statement and answer options in string variables welcome_message = "Welcome to the Personality Quiz!" answer_prompt = "Enter your answer here (1-5): " # Function to extract the statement from a question string def extract_statement(question): return question.split('.', 1)[0] # Initialize an empty list to store user answers user_answers = [] # Print the welcome message print(welcome_message + "\n") # Ask the user all five questions and record their answers for i in range(5): # Get the selected question selected_question = questions[i] # Extract the statement from the selected question question_statement = extract_statement(selected_question) # Print the statement with quotation marks and formatting print(f'How much do you agree with this statement?\n"{question_statement}"\n') # Print answer options print("1. Strongly disagree") print("2. Disagree") print("3. Neutral") print("4. Agree") print("5. Strongly agree\n") # Get the user's answer user_answer = int(input(answer_prompt)) # Validate the user input while user_answer < 1 or user_answer > 5: print("Invalid input. Please enter a number between 1 and 5.") user_answer = int(input(answer_prompt)) # Append the user's answer to the list user_answers.append(user_answer) # Print the user's answers print("\nYour answers are:", user_answers) ------------------------------------------------------------ Given code for THIS Milestone to complete with # TODO: Write your header comment here. import driver # Do not remove this quiz_type = {1:"BabyAnimals", 2:"Brooklyn99", 3:"Disney", 4:"Hogwarts", 5:"MyersBriggs", 6:"SesameStreet", 7:"StarWars", 8:"Vegetables"} questions = ["I am the life of the party. E:1", "I warm up quickly to others. A:1 E:1", "I never at a loss for words. O:1 E:1", "I seldom get lost in thought. C:1 O:-1", "I am easily distracted. N:-1 C:-1"] # COPY AND PASTE YOUR CODE FROM MILESTONE #1 AND MILESTONE #3 HERE # TODO: Write your code here. -------------------------------------------------------------------- Follow all directions and the output code should look EXACTLY like this Welcome to the Personality Quiz! ⏎ What type of Personality Quiz do you want to run? ⏎ 1 - BabyAnimals 2 - Brooklyn99 3 - Disney 4 - Hogwarts 5 - MyersBriggs 6 - SesameStreet 7 - StarWars 8 - Vegetables ⏎ Choose test number (1-8): Great! Let's begin the BabyAnimals Personality Quiz... ⏎ How much do you agree with this statement? "I am the life of the party" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I warm up quickly to others" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I never at a loss for words" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I seldom get lost in thought" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): How much do you agree with this statement? "I am easily distracted" ⏎ 1. Strongly disagree 2. Disagree 3. Neutral 4. Agree 5. Strongly agree ⏎ Enter your answer here (1-5): You got Baby Hamster! ⏎ Goodbye.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 6PE
Related questions
Question
My code from milestone 1
# Define the quiz_type dictionary
quiz_type = {
1: "BabyAnimals",
2: "Brooklyn99",
3: "Disney",
4: "Hogwarts",
5: "MyersBriggs",
6: "SesameStreet",
7: "StarWars",
8: "Vegetables"
}
# Print the welcome message
print("Welcome to the Personality Quiz!")
print()
print("What type of Personality Quiz do you want to run?")
print()
for number, quiz_name in quiz_type.items():
print(f"{number} - {quiz_name}")
print()
test_number = int(input("Choose test number (1-8): "))
# Check if the test_number is valid
if test_number in quiz_type:
quiz_name = quiz_type[test_number]
print()
print(f"Great! Let's begin the {quiz_name} Personality Quiz...")
else:
print("Invalid test number. Please choose a number between 1 and 8.")
-------------------------------------------------------------------
Milestone #3 code :
# Ending statements with the period to easily split them as mentioned in the question.
questions = [
"I am the life of the party. E:1",
"I warm up quickly to others. A:1 E:1",
"I never at a loss for words. O:1 E:1",
"I seldom get lost in thought. C:1 O:-1",
"I am easily distracted. N:-1 C:-1"
]
# Store the printed statement and answer options in string variables
welcome_message = "Welcome to the Personality Quiz!"
answer_prompt = "Enter your answer here (1-5): "
# Function to extract the statement from a question string
def extract_statement(question):
return question.split('.', 1)[0]
# Initialize an empty list to store user answers
user_answers = []
# Print the welcome message
print(welcome_message + "\n")
# Ask the user all five questions and record their answers
for i in range(5):
# Get the selected question
selected_question = questions[i]
# Extract the statement from the selected question
question_statement = extract_statement(selected_question)
# Print the statement with quotation marks and formatting
print(f'How much do you agree with this statement?\n"{question_statement}"\n')
# Print answer options
print("1. Strongly disagree")
print("2. Disagree")
print("3. Neutral")
print("4. Agree")
print("5. Strongly agree\n")
# Get the user's answer
user_answer = int(input(answer_prompt))
# Validate the user input
while user_answer < 1 or user_answer > 5:
print("Invalid input. Please enter a number between 1 and 5.")
user_answer = int(input(answer_prompt))
# Append the user's answer to the list
user_answers.append(user_answer)
# Print the user's answers
print("\nYour answers are:", user_answers)
------------------------------------------------------------
Given code for THIS Milestone to complete with
# TODO: Write your header comment here.
import driver # Do not remove this
quiz_type = {1:"BabyAnimals", 2:"Brooklyn99", 3:"Disney", 4:"Hogwarts", 5:"MyersBriggs", 6:"SesameStreet", 7:"StarWars", 8:"Vegetables"}
questions = ["I am the life of the party. E:1", "I warm up quickly to others. A:1 E:1", "I never at a loss for words. O:1 E:1", "I seldom get lost in thought. C:1 O:-1", "I am easily distracted. N:-1 C:-1"]
# COPY AND PASTE YOUR CODE FROM MILESTONE #1 AND MILESTONE #3 HERE
# TODO: Write your code here.
--------------------------------------------------------------------
Follow all directions and the output code should look EXACTLY like this
Welcome to the Personality Quiz!
⏎
What type of Personality Quiz do you want to run?
⏎
1 - BabyAnimals
2 - Brooklyn99
3 - Disney
4 - Hogwarts
5 - MyersBriggs
6 - SesameStreet
7 - StarWars
8 - Vegetables
⏎
Choose test number (1-8):
Great! Let's begin the BabyAnimals Personality Quiz...
⏎
How much do you agree with this statement?
"I am the life of the party"
⏎
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
⏎
Enter your answer here (1-5):
How much do you agree with this statement?
"I warm up quickly to others"
⏎
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
⏎
Enter your answer here (1-5):
How much do you agree with this statement?
"I never at a loss for words"
⏎
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
⏎
Enter your answer here (1-5):
How much do you agree with this statement?
"I seldom get lost in thought"
⏎
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
⏎
Enter your answer here (1-5):
How much do you agree with this statement?
"I am easily distracted"
⏎
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
⏎
Enter your answer here (1-5):
You got Baby Hamster!
⏎
Goodbye.

Transcribed Image Text:In a file called main.py, implement a program that finds the fictional character who is most similar to the user. The program should have six
input prompts: the integer value of the personality quiz number and the five integer values of the user's answers on five questions.
Your program should combine everything you did in the previous milestones: print welcome message and list of the personality Quizzes,
ask the user for the quiz type, ask five questions, record the user's answers into the list, and then print name of the fictional character who
is most similar to the user based on the user's answers. So copy and paste your fully tested and functional code from Milestone #1 and
Milestone #3. Before writing any new code, make sure that combined code is working correctly. See example below.
Welcome to the Personality Quiz!
What type of Personality Quiz do you want to run?
1- BabyAnimals
2 - Brooklyn99
3- Disney
4- Hogwarts
5- MyersBriggs
6- SesareStreet
7- StarWars
8- Vegetables
Choose test number (1-8): 1
Great! Let's begin the BabyAnimals Personality Quiz...
How much do you agree with this statement?
"I am the life of the party"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): 5
How much do you agree with this statement?
"I an easily distracted"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): 1
You got Baby Hamster!
Goodbye.
You do not need to write any code to find the closest match to the user. We wrote it for you in drive gy file. At this point, you have the
questions list, the list of users answers and the personality quiz file name. All you left to do is to find a function in drivargy which takes
these things as parameters and returns a name of the fictional character who is most similar to the user.
Here's an example to how to call the function from driver.py:
import driver
a driver.find_match(b, c, d)
If you look in drivergy, there is a function called "find-match and in the function comment, it describes what a, b, c, dare, a is what is
returned from the function (a string, the match you are looking for). The variables b, c, and d are described in the comment. You already
have all three in the code you have previously written. There isn't too much to do with this milestone, just write the line above with the
proper variables specific to your code and then print out the result.
At the end of the semester, you should be able to fully understand all the code in driver.py. But right now, it is probably confusing!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
You were supposed to use import driver command and either way it doesn't work look at your output and expected output...
THESE ARE WITH INPUTS 1 5 4 3 2 1
Yours:
Welcome to the Personality Quiz!
What type of Personality Quiz do you want to run?
1 - BabyAnimals
2 - Brooklyn99
3 - Disney
4 - Hogwarts
5 - MyersBriggs
6 - SesameStreet
7 - StarWars
8 - Vegetables
Choose test number (1-8):
Great! Let's begin the BabyAnimals Personality Quiz...
How much do you agree with this statement?
"I am the life of the party"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): How much do you agree with this statement?
"I warm up quickly to others"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): How much do you agree with this statement?
"I never at a loss for words"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): How much do you agree with this statement?
"I seldom get lost in thought"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5): How much do you agree with this statement?
"I am easily distracted"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
You got Baby Hamster!
Goodbye.
Expected
Welcome to the Personality Quiz!
What type of Personality Quiz do you want to run?
1 - BabyAnimals
2 - Brooklyn99
3 - Disney
4 - Hogwarts
5 - MyersBriggs
6 - SesameStreet
7 - StarWars
8 - Vegetables
Choose test number (1-8):
Great! Let's begin the BabyAnimals Personality Quiz...
⏎
How much do you agree with this statement?
"I am the life of the party"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
How much do you agree with this statement?
"I warm up quickly to others"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
How much do you agree with this statement?
"I never at a loss for words"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
How much do you agree with this statement?
"I seldom get lost in thought"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
How much do you agree with this statement?
"I am easily distracted"
1. Strongly disagree
2. Disagree
3. Neutral
4. Agree
5. Strongly agree
Enter your answer here (1-5):
You got Baby Hamster!
Goodbye.
--------------------------------------------------------------
It is wrong for most inputs too
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
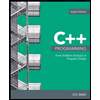
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
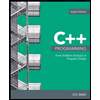
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning