Your program should use a loop to read data from a text file which contains students' names and 3 exam scores. The program should calculate the exam average for each student, determine the appropriate letter grade for that exam average, and print the name, average, and letter grade. After printing all this, you need to print the count of the total number of A's, B's, C's, D's, and F's for the whole class. The text file you need to use can be accessed using this link: scores.txt. Copy this file to the folder where your program will reside. The text file contains a list of 30 records, and each record contains a name and that person's 3 test scores. Each field is separated by a tab character. The first 2 records looks like this: Davis 88 92 84Wilson 98 80 73 and so on. Your program will need to open this file and read each line until the end of file. I recommend calculating a student's exam average and letter grade as you read the file, although this is not a strict requirement. Once you've calculated the exam average from the 3 scores for a student, like student Davis or Wilson above, you can find their letter grade using the following grading scale. 90 - 100 A80 - 89 B70 - 79 C60 - 69 D< 60 F So, your output should look something like the following, but with all 30 students and an accurate count for the number of A's, B's, C's, D's, and F's. There may or may not be 4 A's and 8 B's. To get full credit your program needs to accomplish the following. You need to print each student's name, exam average, and letter grade based on the averages of their 3 exam scores. After printing all 30 of the names, averages, and grades, you need to print a summary stating the number of the number of A's, B's, C's, D's, and F's for the whole class. To get the letter grades you'll need to calculate the average of the 3 exam for each student and determine the appropriate letter grade for that average based on the criteria listed above. The code that determines the letter grade needs to be in it's own function. You need to process the data with a loop, and that loop should be able to work properly even if the number of students in the file varies from day to day. That is, don't use 30 as a magic number. The program should work no matter how many records the file might contain. Your program must have at least 2 functions written by you. A main() function, and a function to determine the letter grade. You could easily have more than 2 functions if you choose, but you need 2 minimum. The non-main function should require passing an argument (or augments) or returning a value, or both. Knowing how to do arguments/return values is a goal of the exercise. Also, it is time we used a little formatting. Use formatting to have the names and grades all line up in neat columns. That is, the letter grades should print in their own straight column, where the grade's printed position does not vary with the length of the name. My source codedef main():def main(): #set count of gradesreadFile()#readFile reads text file scores.txt and print the name,grade and count of each gradedef readFile():name=""gradeA=0gradeB=0gradeC=0gradeD=0gradeF=0avg=0print('Name','Average','Grade')print("-"*30)studFile=open('scores.txt','r')for line in studFile.readlines():val=line.split()avg=(float(val[1])+float(val[2])+float(val[3]))/3.0grade=getGrade(avg)#count grades of each letterif(grade=='A'):gradeA=gradeA+1elif(grade=='B'):gradeB=gradeB+1elif(grade=='C'):gradeC=gradeC+1elif(grade=='D'):gradeD=gradeD+1elif(grade=='F'):gradeF=gradeF+1print(val[0], avg, grade)#print gradesprint('Number of A''s:', gradeA)print('Number of B''s:', gradeB)print('Number of C''s:', gradeC)print('Number of D''s:', gradeD)print('Number of F''s:', gradeF)#Returns character gradedef getGrade(avg):grade=' '#Set grade on average scoreif(avg>=90):grade='A'elif avg>=80 and avg<90:grade='B'elif avg>=70 and avg<80:grade='C'elif avg>=60 and avg<70:grade='D'else:grade='F'return grademain()
Your program should use a loop to read data from a text file which contains students' names and 3 exam scores. The program should calculate the exam average for each student, determine the appropriate letter grade for that exam average, and print the name, average, and letter grade. After printing all this, you need to print the count of the total number of A's, B's, C's, D's, and F's for the whole class.
The text file you need to use can be accessed using this link: scores.txt.
Copy this file to the folder where your program will reside. The text file contains a list of 30 records, and each record contains a name and that person's 3 test scores. Each field is separated by a tab character. The first 2 records looks like this:
Davis 88 92 84
Wilson 98 80 73
and so on. Your program will need to open this file and read each line until the end of file. I recommend calculating a student's exam average and letter grade as you read the file, although this is not a strict requirement.
Once you've calculated the exam average from the 3 scores for a student, like student Davis or Wilson above, you can find their letter grade using the following grading scale.
90 - 100 A
80 - 89 B
70 - 79 C
60 - 69 D
< 60 F
So, your output should look something like the following, but with all 30 students and an accurate count for the number of A's, B's, C's, D's, and F's. There may or may not be 4 A's and 8 B's.
To get full credit your program needs to accomplish the following.
- You need to print each student's name, exam average, and letter grade based on the averages of their 3 exam scores.
- After printing all 30 of the names, averages, and grades, you need to print a summary stating the number of the number of A's, B's, C's, D's, and F's for the whole class.
- To get the letter grades you'll need to calculate the average of the 3 exam for each student and determine the appropriate letter grade for that average based on the criteria listed above. The code that determines the letter grade needs to be in it's own function.
- You need to process the data with a loop, and that loop should be able to work properly even if the number of students in the file varies from day to day. That is, don't use 30 as a magic number. The program should work no matter how many records the file might contain.
- Your program must have at least 2 functions written by you. A main() function, and a function to determine the letter grade. You could easily have more than 2 functions if you choose, but you need 2 minimum. The non-main function should require passing an argument (or augments) or returning a value, or both. Knowing how to do arguments/return values is a goal of the exercise.
- Also, it is time we used a little formatting. Use formatting to have the names and grades all line up in neat columns. That is, the letter grades should print in their own straight column, where the grade's printed position does not vary with the length of the name.
- My source codedef main():
def main(): - #set count of grades
readFile()
#readFile reads text file scores.txt and print the name,grade and count of each grade
def readFile():
name=""
gradeA=0
gradeB=0
gradeC=0
gradeD=0
gradeF=0
avg=0
print('Name','Average','Grade')
print("-"*30)
studFile=open('scores.txt','r')
for line in studFile.readlines():
val=line.split()
avg=(float(val[1])+float(val[2])+float(val[3]))/3.0
grade=getGrade(avg)
#count grades of each letter
if(grade=='A'):
gradeA=gradeA+1
elif(grade=='B'):
gradeB=gradeB+1
elif(grade=='C'):
gradeC=gradeC+1
elif(grade=='D'):
gradeD=gradeD+1
elif(grade=='F'):
gradeF=gradeF+1
print(val[0], avg, grade)
#print grades
print('Number of A''s:', gradeA)
print('Number of B''s:', gradeB)
print('Number of C''s:', gradeC)
print('Number of D''s:', gradeD)
print('Number of F''s:', gradeF)
#Returns character grade
def getGrade(avg):
grade=' '
#Set grade on average score
if(avg>=90):
grade='A'
elif avg>=80 and avg<90:
grade='B'
elif avg>=70 and avg<80:
grade='C'
elif avg>=60 and avg<70:
grade='D'
else:
grade='F'
return grade
main()

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

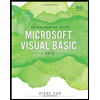
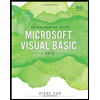