ython - This is my code for a baby bitcoin program (question is below the code) class BitCoin: bcoins = 0 balance = 0 n = 0 def setBitCoin(self): self.bcoins=self.bcoins+0.5 self.balance=self.balance-0.5*n print("Balance after buying 0.5 bitcoin") print("The amount left in USD: ",self.balance) print("The number of bitcoins in the wallet: ",self.bcoins) print("The USD value of the bitcoins: ",self.bcoins*self.n) def getValue(self): self.balance=self.balance-3000 self.bcoins=self.bcoins+3000/self.n print("Balance after buying bitcoin of worth 3000 USD") print("The amount left in USD: ",self.balance) print("The number of bitcoins in the wallet: ",self.bcoins) print("The USD value of the bitcoins: ",self.bcoins*self.n) def sellCoin(self): self.bcoins=self.bcoins-0.5 self.balance=self.balance+0.5*self.n print("Balance after selling 0.5 bitcoin") print("The amount left in USD: ",self.balance) print("The number of bitcoins in the wallet: ",self.bcoins) print("The USD value of the bitcoins: ",self.bcoins*self.n) def sell(self): self.bcoins=self.bcoins-3000/self.n self.balance=self.balance+3000 print("Balance after selling bitcoin of worth 3000 USD") print("The amount left in USD: ",self.balance) print("The number of bitcoins in the wallet: ",self.bcoins) print("The USD value of the bitcoins: ",self.bcoins*self.n) balance=int(input("Enter the balance amount in USD: ")) n=int(input("Enter the value of 1 bitcoin in USD: ")) bcoins = 0 b = BitCoin() b.balance = balance b.bcoins = bcoins b.n = n while True: print("1. buy 0.5") print("2. buy 3000") print("3. sell 0.5") print("4. sell 3000") option = int(input("Enter choice:")) if option==1: bcoins = b.setBitCoin() elif option==2: bcoins = b.getValue() elif option==3: bcoins = b.sellCoin() elif option==4: bcoins = b.sell() else: break print("Final Balance") print("The amount left in USD: ",BitCoin.balance) print("The number of bitcoins in the wallet: ",BitCoin.bcoins) print("The USD value of the bitcoins: ",BitCoin.bcoins*BitCoin.n) Problem: Make a class called ledger which does the following Need a class that is going to turn transactions into a string and store them for the history. 1) It will store the transaction strings just so we have a record of the buy & sell commands 2) It will be able to print out the transaction history if requested. Using list or dict.
Python - This is my code for a baby bitcoin program (question is below the code)
class BitCoin:
bcoins = 0
balance = 0
n = 0
def setBitCoin(self):
self.bcoins=self.bcoins+0.5
self.balance=self.balance-0.5*n
print("Balance after buying 0.5 bitcoin")
print("The amount left in USD: ",self.balance)
print("The number of bitcoins in the wallet: ",self.bcoins)
print("The USD value of the bitcoins: ",self.bcoins*self.n)
def getValue(self):
self.balance=self.balance-3000
self.bcoins=self.bcoins+3000/self.n
print("Balance after buying bitcoin of worth 3000 USD")
print("The amount left in USD: ",self.balance)
print("The number of bitcoins in the wallet: ",self.bcoins)
print("The USD value of the bitcoins: ",self.bcoins*self.n)
def sellCoin(self):
self.bcoins=self.bcoins-0.5
self.balance=self.balance+0.5*self.n
print("Balance after selling 0.5 bitcoin")
print("The amount left in USD: ",self.balance)
print("The number of bitcoins in the wallet: ",self.bcoins)
print("The USD value of the bitcoins: ",self.bcoins*self.n)
def sell(self):
self.bcoins=self.bcoins-3000/self.n
self.balance=self.balance+3000
print("Balance after selling bitcoin of worth 3000 USD")
print("The amount left in USD: ",self.balance)
print("The number of bitcoins in the wallet: ",self.bcoins)
print("The USD value of the bitcoins: ",self.bcoins*self.n)
balance=int(input("Enter the balance amount in USD: "))
n=int(input("Enter the value of 1 bitcoin in USD: "))
bcoins = 0
b = BitCoin()
b.balance = balance
b.bcoins = bcoins
b.n = n
while True:
print("1. buy 0.5")
print("2. buy 3000")
print("3. sell 0.5")
print("4. sell 3000")
option = int(input("Enter choice:"))
if option==1:
bcoins = b.setBitCoin()
elif option==2:
bcoins = b.getValue()
elif option==3:
bcoins = b.sellCoin()
elif option==4:
bcoins = b.sell()
else:
break
print("Final Balance")
print("The amount left in USD: ",BitCoin.balance)
print("The number of bitcoins in the wallet: ",BitCoin.bcoins)
print("The USD value of the bitcoins: ",BitCoin.bcoins*BitCoin.n)
Problem: Make a class called ledger which does the following
Need a class that is going to turn transactions into a string and store them for the history.
1) It will store the transaction strings just so we have a record of the buy & sell commands 2) It will be able to print out the transaction history if requested. Using list or dict.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

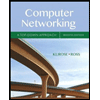
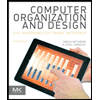
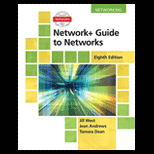
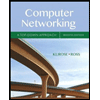
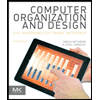
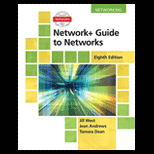
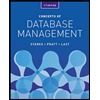
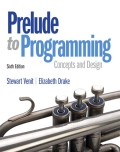
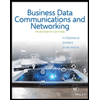