Complete the Course class by implementing the drop_student() instance method, which removes a student (by last name) from the course roster. If the student is not found in the course roster, no student should be dropped. The file main.py contains: • The main function for testing the program. • Class Course represents a course, which contains a list of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three attributes: first name, last name, and GPA. (Hint: get_last() returns the last name field.) Note: For testing purposes, different student values will be used. Ex. For the following students: Henry Nguyen 3.5 Brenda Stern 2.0 Lynda Robison 3.2 Sonya King 3.9 the output for dropping Stern is: Course size: 4 students Course size after drop: 3 students
python
class Student:
def __init__(self, first, last, gpa):
self.first = first # first name
self.last = last # last name
self.gpa = gpa # grade point average
def get_gpa(self):
return self.gpa
def get_last(self):
return self.last
def to_string(self):
return self.first + ' ' + self.last + ' (GPA: ' + str(self.gpa) + ')'
class Course:
def __init__(self):
self.roster = [] # list of Student objects
def drop_student(self, student):
# Type your code here
def add_student(self, student):
self.roster.append(student)
def count_students(self):
return len(self.roster)
if __name__ == "__main__":
course = Course()
course.add_student(Student('Henry', 'Nguyen', 3.5))
course.add_student(Student('Brenda', 'Stern', 2.0))
course.add_student(Student('Lynda', 'Robinson', 3.2))
course.add_student(Student('Sonya', 'King', 3.9))
print('Course size:', course.count_students(),'students')
course.drop_student('Stern')
print('Course size after drop:', course.count_students(),'students')


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

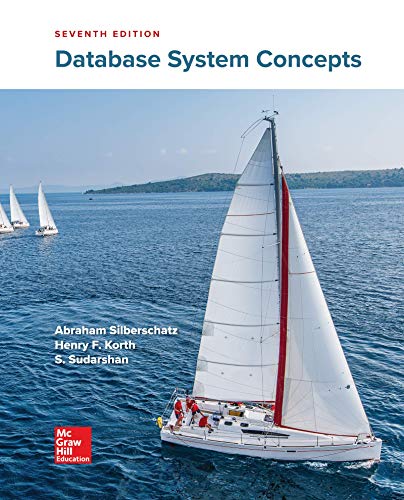
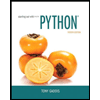
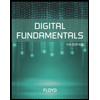
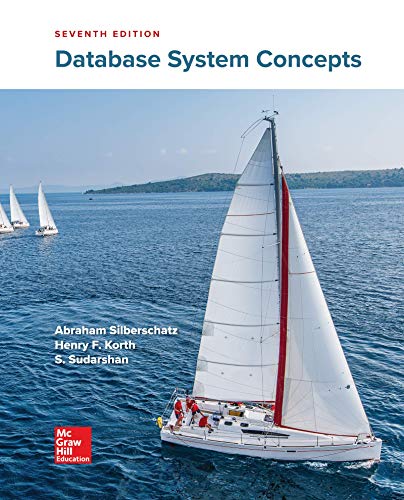
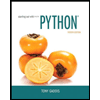
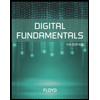
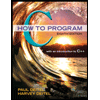
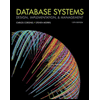
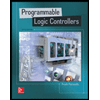