#include #include #include #include #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP #define INIT -127 #define UNDERFLOW (0x80 + 0x02) #define OVERFLOW 0x80+ 0x01 #define BADPTR (0x80+ 0x03) #define CONSUMER_TERMINATION PROBABILITY 40 #define PRODUCER_TERMINATION_PROBABILITY 30 // =========== //sem_t ACCESS; // use a mutex instead of a binary semaphore pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER; sem_t *FULLSPOTS; sem_t *EMPTYSPOTS; struct timespec remaining; struct timespec requested_time; struct Queue{- void enqueue(struct Queue *q, char x)- char dequeue (struct Queue *q) - wvoid build(struct Queue **q, unsigned int length) - 1/ ========== void *producer(void *qptr ) --- You can modify these two constants for test and experimentation woid *consumer (void *qptr) --- void closeup (void); int main(int argc, const char * argv[])- ======== LOCKED ========== UNLOCKED ================ Don't modify this code segment
#include #include #include #include #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP #define INIT -127 #define UNDERFLOW (0x80 + 0x02) #define OVERFLOW 0x80+ 0x01 #define BADPTR (0x80+ 0x03) #define CONSUMER_TERMINATION PROBABILITY 40 #define PRODUCER_TERMINATION_PROBABILITY 30 // =========== //sem_t ACCESS; // use a mutex instead of a binary semaphore pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER; sem_t *FULLSPOTS; sem_t *EMPTYSPOTS; struct timespec remaining; struct timespec requested_time; struct Queue{- void enqueue(struct Queue *q, char x)- char dequeue (struct Queue *q) - wvoid build(struct Queue **q, unsigned int length) - 1/ ========== void *producer(void *qptr ) --- You can modify these two constants for test and experimentation woid *consumer (void *qptr) --- void closeup (void); int main(int argc, const char * argv[])- ======== LOCKED ========== UNLOCKED ================ Don't modify this code segment
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.1: Addresses And Pointers
Problem 5E
Related questions
Question
100%
Complete the following code. The goal is to implement the producer-consumer problem. You are expected to extend the provided C code to synchronize the thread operations consumer() and producer() such that an underflow and overflow of the queue is prevented. You are not allowed to change the code for implementing the queue operations, that is the code between lines 25 and 126 as shown in the Figure below. You must complete the missing parts between lines 226-261 as shown in the screenshot.
![ܕ : ܘܢܬܬܢ ܪ ܪ ܪ ܪ . ܝ . . ܫ ܕ
13
#include <pthread.h>
14 #include <semaphore.h>
15 #include <errno.h>
16 #include <fcntl.h>
17 #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP
18
#define INIT -127
19 #define UNDERFLOW (0x80 + 0x02)
20 #define OVERFLOW 0x80+ 0x01
21 #define BADPTR (0x80 + 0x03)
22 #define CONSUMER_TERMINATION_PROBABILITY 40
23 #define PRODUCER_TERMINATION PROBABILITY 30
24
25 //
26
27
28 sem_t *FULLSPOTS;
29 sem_t *EMPTYSPOTS;
You can modify these two constants
for test and experimentation
//sem_t ACCESS; // use a mutex instead of a binary semaphore
pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER;
30 struct timespec remaining;
31 struct timespec requested_time;
32 > struct Queue{---
38
39
40> void enqueue (struct Queue *q, char x) -
65 > char dequeue(struct Queue *q) -
97 > void build(struct Queue **q, unsigned int length) ---
125
126 //
127
128 void *producer ( void *qptr ) ---
179
180 void *consumer( void *qptr) ...
225
226 void closeup (void);
227 int main(int argc, const char * argv[])--
308
309> void closeup (void) ...
LOCKED
UNLOCKED
Don't modify this code segment
TOTAL](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff9be1d69-a222-4ca1-8ade-d83f4256e548%2Fd7df4cf5-e557-4bfb-af1c-f6cefeeafaac%2Fpptl0bf_processed.png&w=3840&q=75)
Transcribed Image Text:ܕ : ܘܢܬܬܢ ܪ ܪ ܪ ܪ . ܝ . . ܫ ܕ
13
#include <pthread.h>
14 #include <semaphore.h>
15 #include <errno.h>
16 #include <fcntl.h>
17 #define MAX_LENGTH_CAP 100 You can modify MAX_LENGTH_CAP
18
#define INIT -127
19 #define UNDERFLOW (0x80 + 0x02)
20 #define OVERFLOW 0x80+ 0x01
21 #define BADPTR (0x80 + 0x03)
22 #define CONSUMER_TERMINATION_PROBABILITY 40
23 #define PRODUCER_TERMINATION PROBABILITY 30
24
25 //
26
27
28 sem_t *FULLSPOTS;
29 sem_t *EMPTYSPOTS;
You can modify these two constants
for test and experimentation
//sem_t ACCESS; // use a mutex instead of a binary semaphore
pthread_mutex_t ACCESS = PTHREAD_MUTEX_INITIALIZER;
30 struct timespec remaining;
31 struct timespec requested_time;
32 > struct Queue{---
38
39
40> void enqueue (struct Queue *q, char x) -
65 > char dequeue(struct Queue *q) -
97 > void build(struct Queue **q, unsigned int length) ---
125
126 //
127
128 void *producer ( void *qptr ) ---
179
180 void *consumer( void *qptr) ...
225
226 void closeup (void);
227 int main(int argc, const char * argv[])--
308
309> void closeup (void) ...
LOCKED
UNLOCKED
Don't modify this code segment
TOTAL
![f
225
226 void closeup (void);
227 int main(int argc, const char * argv[])
228 {
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
pthread_t thread [6]; // declares the identifier for the thread we will create.
pthread_attr_t attr;
int iret [6];
int q_test_size = 5;
struct Queue *qptr = NULL;
build (&qptr, q_test_size);
(&attr);
closeup();
FULLSPOTS = (sem_t*) sem_open ("/FULLSPOTS", O_CREAT 10_EXCL, 0644,
if (
}
perror("sem_open");
exit (EXIT_FAILURE);
EMPTYSPOTS = sem_open("/EMPTYSPOTS", O_CREATIO_EXCL, 0644,
if
perror("sem_open");
exit (EXIT_FAILURE);
}
int x=0;
for(; x<5; x++) {
iret [x] = pthread_create(&thread [x], &attr, producer, qptr); // creat 5 producers
if (!iret [x]) // check for success
printf("PRODUCER_%d was created successfully.", x);
}
iret [x] = pthread_create(&thread [x], &attr, consumer, qptr); // ctreate one consumer
if (!iret [x])
printf("CONSUMER_%d was created successfully.", x);
for(int x=0; x<6; x++) // Wait for all threads; verify the for-loop
closeup ();
if (qptr->data) free(qptr->data);
if(qptr) free (qptr);
264
265
266
267
268
269> void closeup (void) --
printf("\n");
return 0;
& main
Ⓡ⁰ A⁰
Figure 2 You must complete the missing parts. between lines 226 - 261 as shown in this screenshot
> TOTAL](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff9be1d69-a222-4ca1-8ade-d83f4256e548%2Fd7df4cf5-e557-4bfb-af1c-f6cefeeafaac%2F7k3ogpn_processed.png&w=3840&q=75)
Transcribed Image Text:f
225
226 void closeup (void);
227 int main(int argc, const char * argv[])
228 {
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
pthread_t thread [6]; // declares the identifier for the thread we will create.
pthread_attr_t attr;
int iret [6];
int q_test_size = 5;
struct Queue *qptr = NULL;
build (&qptr, q_test_size);
(&attr);
closeup();
FULLSPOTS = (sem_t*) sem_open ("/FULLSPOTS", O_CREAT 10_EXCL, 0644,
if (
}
perror("sem_open");
exit (EXIT_FAILURE);
EMPTYSPOTS = sem_open("/EMPTYSPOTS", O_CREATIO_EXCL, 0644,
if
perror("sem_open");
exit (EXIT_FAILURE);
}
int x=0;
for(; x<5; x++) {
iret [x] = pthread_create(&thread [x], &attr, producer, qptr); // creat 5 producers
if (!iret [x]) // check for success
printf("PRODUCER_%d was created successfully.", x);
}
iret [x] = pthread_create(&thread [x], &attr, consumer, qptr); // ctreate one consumer
if (!iret [x])
printf("CONSUMER_%d was created successfully.", x);
for(int x=0; x<6; x++) // Wait for all threads; verify the for-loop
closeup ();
if (qptr->data) free(qptr->data);
if(qptr) free (qptr);
264
265
266
267
268
269> void closeup (void) --
printf("\n");
return 0;
& main
Ⓡ⁰ A⁰
Figure 2 You must complete the missing parts. between lines 226 - 261 as shown in this screenshot
> TOTAL
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
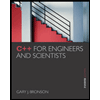
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
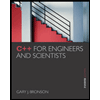
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr