1. Make a function called fileSort(). fileSort() should take in three parameters which will correspond to arguments in the following order from left to right: a. The name of a .csv file to open and read in the contents. b. The name of a .csv file to open and write out to. c. A flag which specifies what your function should do with the contents of the .csv file.
1. Make a function called fileSort(). fileSort() should take in three parameters which will correspond to arguments in the following order from left to right: a. The name of a .csv file to open and read in the contents. b. The name of a .csv file to open and write out to. c. A flag which specifies what your function should do with the contents of the .csv file.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.3: Random File Access
Problem 6E
Related questions
Question
python please help no other answers have been right :(
![1. Make a function called fileSort(). fileSort() should take in three parameters which will
correspond to arguments in the following order from left to right:
a. The name of a .csv file to open and read in the contents.
b. The name of a .csv file to open and write out to.
c. A flag which specifies what your function should do with the contents of the .csv
file.
2. Assume that the contents of the .csv file you are reading from contains records which all
contain 3 elements e.g. the .csv has 3 columns. Also assume there is NO HEADER on the
input file. You should start by reading the contents of the .csv file into a list of lists. Your
job then is to write a custom sorting algorithm that will sort ALL the records based on
the value at one position in each record based on the flag (third parameter).
a. If the third parameter is equal to 0 then you should sort the list of lists based on
the value of at the 0th index of each record (list element). Assume it is an int.
b.
If the third parameter is equal to 1 then you should sort the list of lists based on
the value of at the 1st index of each record (list element). Assume it is an int.
c. If the third parameter is equal to 2 then you should sort the list of lists based on
the value of at the 2nd index of each record (list element). Assume it is an int.
3. For example: Lets say your csv file contained the following records: [cats, dogs, turtles],
[turtles, cats, dogs], [dogs,turtles, cats]. If your third parameter was 2 then you should
produce the following sorted list of lists: [dogs,turtles,cats], [turtles, cats, dogs],
[cats, dogs,turtles]. Note that the contents of the lists (records) are not changing in
terms of the ordering of the elements, rather the lists themselves are being rearranged
in the larger list.
4. Now: Write out your newly sorted list of lists to the file specified in your second
parameter. Write in "write" mode not "append" mode. Your function does not need to
return anything.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1f508c53-af29-498e-8791-77e45ebbdaaf%2Fcec65b5d-74ac-44ea-b3c6-980bd15f6e9f%2Fajzkiov_processed.png&w=3840&q=75)
Transcribed Image Text:1. Make a function called fileSort(). fileSort() should take in three parameters which will
correspond to arguments in the following order from left to right:
a. The name of a .csv file to open and read in the contents.
b. The name of a .csv file to open and write out to.
c. A flag which specifies what your function should do with the contents of the .csv
file.
2. Assume that the contents of the .csv file you are reading from contains records which all
contain 3 elements e.g. the .csv has 3 columns. Also assume there is NO HEADER on the
input file. You should start by reading the contents of the .csv file into a list of lists. Your
job then is to write a custom sorting algorithm that will sort ALL the records based on
the value at one position in each record based on the flag (third parameter).
a. If the third parameter is equal to 0 then you should sort the list of lists based on
the value of at the 0th index of each record (list element). Assume it is an int.
b.
If the third parameter is equal to 1 then you should sort the list of lists based on
the value of at the 1st index of each record (list element). Assume it is an int.
c. If the third parameter is equal to 2 then you should sort the list of lists based on
the value of at the 2nd index of each record (list element). Assume it is an int.
3. For example: Lets say your csv file contained the following records: [cats, dogs, turtles],
[turtles, cats, dogs], [dogs,turtles, cats]. If your third parameter was 2 then you should
produce the following sorted list of lists: [dogs,turtles,cats], [turtles, cats, dogs],
[cats, dogs,turtles]. Note that the contents of the lists (records) are not changing in
terms of the ordering of the elements, rather the lists themselves are being rearranged
in the larger list.
4. Now: Write out your newly sorted list of lists to the file specified in your second
parameter. Write in "write" mode not "append" mode. Your function does not need to
return anything.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
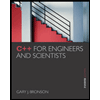
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
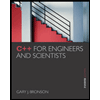
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr