Below you can see a Mealy and Moore design code as well as simulation (test bench) code. Can you write note on side of code illustrating what the line represents and explain how the two codes are behaving differently? Mealy: Design source code: module mealy #Example of where the notes should go to represent how the lines are behaving ( input shift_start, input shift_stop, input rst, input clk, input d, output reg [7:0] q ); parameter Idle =2'd0; parameter Start =2'd1; parameter Run =2'd2; parameter Stop =2'd3; reg [1:0] state; reg [4:0] delay_cnt; always @(posedge clk or negedge rst) begin if(!rst) begin state <= Idle; delay_cnt <= 0; q <= 0; end else case(state) Idle : begin if(shift_start) state <= Start; end Start : begin if(delay_cnt ==5'd99) begin delay_cnt <= 0; state <= Run; end else delay_cnt <= delay_cnt + 1'b1; end Run : begin if(shift_stop) state <= Stop; else q <= {q[6:0], d}; end Stop : begin q <= 0; state <= Idle; end default: state <= Idle; endcase end endmodule Simulation code: module fsm_tb(); reg shift_start; reg shift_stop; reg rst; reg clk; reg d; wire [7:0] q; initial begin rst = 0; clk = 0; d = 0; #200 rst = 1; forever begin #({$random}%100) d = ~d; end end initial begin shift_start = 0; shift_stop = 0; #300 shift_start = 1; #1000 shift_start = 0; shift_stop = 1; #50 shift_start = 0; end always #10 clk = ~ clk; mealy t0 ( .shift_start(shift_start), .shift_stop(shift_stop), .rst(rst), .clk(clk), .d(d), .q(q) ); endmodule Moore design code: module fsm_moore ( input shift_start, input shift_stop, input rst, input clk, input d, output reg [7:0] q ); parameter Idle = 2'd0 ; //Idle state parameter Start = 2'd1 ; //Start state parameter Run = 2'd2 ; //Run state parameter Stop = 2'd3 ; //Stop state reg [1:0] current_state ; //statement reg [1:0] next_state ; reg [4:0] delay_cnt ; //delay counter //First part: statement transition always @(posedge clk or negedge rst) begin if (!rst) current_state <= Idle ; else current_state <= next_state ; end //Second part: combination logic, judge statement transition always @(*) begin case(current_state) Idle : begin if (shift_start) next_state <= Start ; else next_state <= Idle ; end Start : begin if (delay_cnt == 5'd99) next_state <= Run ; else next_state <= Start ; end Run : begin if (shift_stop) next_state <= Stop ; else next_state <= Run ; end Stop : next_state <= Idle ; default: next_state <= Idle ; endcase end //Last part: output data always @(posedge clk or negedge rst) begin if (!rst) delay_cnt <= 0 ; else if (current_state == Start) delay_cnt <= delay_cnt + 1'b1 ; else delay_cnt <= 0 ; end always @(posedge clk or negedge rst) begin if (!rst) q <= 0 ; else if (current_state == Run) q <= {q[6:0], d} ; else q <= 0 ; end endmodule Simulation: module moore_tb(); reg shift_start; reg shift_stop; reg rst; reg clk; reg d; wire [7:0] q; initial begin rst = 0; clk = 0; d = 0; #200 rst = 1; forever begin #({$random}%100) d = ~d; end end initial begin shift_start = 0; shift_stop = 0; #300 shift_start = 1; #1000 shift_start = 0; shift_stop = 1; #50 shift_start = 0; end always #10 clk = ~ clk; mealy t0 ( .shift_start(shift_start), .shift_stop(shift_stop), .rst(rst), .clk(clk), .d(d), .q(q) ); endmodule
Below you can see a Mealy and Moore design code as well as simulation (test bench) code.
Can you write note on side of code illustrating what the line represents and explain how the two codes are behaving differently?
Mealy:
Design source code:
module mealy #Example of where the notes should go to represent how the lines are behaving
(
input shift_start,
input shift_stop,
input rst,
input clk,
input d,
output reg [7:0] q
);
parameter Idle =2'd0;
parameter Start =2'd1;
parameter Run =2'd2;
parameter Stop =2'd3;
reg [1:0] state;
reg [4:0] delay_cnt;
always @(posedge clk or negedge rst)
begin
if(!rst)
begin
state <= Idle;
delay_cnt <= 0;
q <= 0;
end
else
case(state)
Idle : begin
if(shift_start)
state <= Start;
end
Start : begin
if(delay_cnt ==5'd99)
begin
delay_cnt <= 0;
state <= Run;
end
else
delay_cnt <= delay_cnt + 1'b1;
end
Run : begin
if(shift_stop)
state <= Stop;
else
q <= {q[6:0], d};
end
Stop : begin
q <= 0;
state <= Idle;
end
default: state <= Idle;
endcase
end
endmodule
Simulation code:
module fsm_tb();
reg shift_start;
reg shift_stop;
reg rst;
reg clk;
reg d;
wire [7:0] q;
initial
begin
rst = 0;
clk = 0;
d = 0;
#200 rst = 1;
forever
begin
#({$random}%100)
d = ~d;
end
end
initial
begin
shift_start = 0;
shift_stop = 0;
#300 shift_start = 1;
#1000 shift_start = 0;
shift_stop = 1;
#50 shift_start = 0;
end
always #10 clk = ~ clk;
mealy t0
(
.shift_start(shift_start),
.shift_stop(shift_stop),
.rst(rst),
.clk(clk),
.d(d),
.q(q)
);
endmodule
Moore design code:
module fsm_moore
(
input shift_start,
input shift_stop,
input rst,
input clk,
input d,
output reg [7:0] q
);
parameter Idle = 2'd0 ; //Idle state
parameter Start = 2'd1 ; //Start state
parameter Run = 2'd2 ; //Run state
parameter Stop = 2'd3 ; //Stop state
reg [1:0] current_state ; //statement
reg [1:0] next_state ;
reg [4:0] delay_cnt ; //delay counter
//First part: statement transition
always @(posedge clk or negedge rst)
begin
if (!rst)
current_state <= Idle ;
else
current_state <= next_state ;
end
//Second part: combination logic, judge statement transition
always @(*)
begin
case(current_state)
Idle : begin
if (shift_start)
next_state <= Start ;
else
next_state <= Idle ;
end
Start : begin
if (delay_cnt == 5'd99)
next_state <= Run ;
else
next_state <= Start ;
end
Run : begin
if (shift_stop)
next_state <= Stop ;
else
next_state <= Run ;
end
Stop : next_state <= Idle ;
default: next_state <= Idle ;
endcase
end
//Last part: output data
always @(posedge clk or negedge rst)
begin
if (!rst)
delay_cnt <= 0 ;
else if (current_state == Start)
delay_cnt <= delay_cnt + 1'b1 ;
else
delay_cnt <= 0 ;
end
always @(posedge clk or negedge rst)
begin
if (!rst)
q <= 0 ;
else if (current_state == Run)
q <= {q[6:0], d} ;
else
q <= 0 ;
end
endmodule
Simulation:
module moore_tb();
reg shift_start;
reg shift_stop;
reg rst;
reg clk;
reg d;
wire [7:0] q;
initial
begin
rst = 0;
clk = 0;
d = 0;
#200 rst = 1;
forever
begin
#({$random}%100)
d = ~d;
end
end
initial
begin
shift_start = 0;
shift_stop = 0;
#300 shift_start = 1;
#1000 shift_start = 0;
shift_stop = 1;
#50 shift_start = 0;
end
always #10 clk = ~ clk;
mealy t0
(
.shift_start(shift_start),
.shift_stop(shift_stop),
.rst(rst),
.clk(clk),
.d(d),
.q(q)
);
endmodule

Step by step
Solved in 4 steps

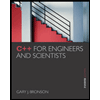
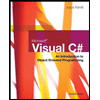
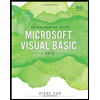
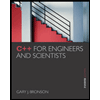
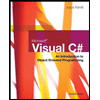
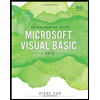
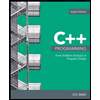