class WaterSort { Character top = null; // create constants for colors static Character red= new Character('r'); static Character blue = new Character('b'); static Character yellow= new Character('g'); // Bottles declaration public static void showAll( StackAsMyArrayList bottles[]) { for (int i = 0; i<=4; i++) { System.out.println("Bottle "+ i+ ": " + bottles[i]); } } public static void main(String args[]) { //part 1 //create the bottle StackAsMyArrayList bottleONE = new StackAsMyArrayList(); System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.push(red); bottleONE.push(red); bottleONE.push(red); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.push(blue); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); bottleONE.pop(); bottleONE.pop(); System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform()); // Bottles declaration // Part 2 // Bottles declaration StackAsMyArrayList bottles[] = new StackAsMyArrayList[5]; //You can do this with a for also bottles[0] = new StackAsMyArrayList(); bottles[1] = new StackAsMyArrayList(); bottles[2] = new StackAsMyArrayList(); bottles[3] = new StackAsMyArrayList(); bottles[4] = new StackAsMyArrayList(); // Fill 3 bottles with specific colors // need not be uniform colours - just easy to do it with a for for (int i = 0; i<4; i++) { bottles[0].push(red); bottles[1].push(blue); bottles[2].push(yellow); bottles[3].push(yellow); bottles[4].push(blue); } // show all bottles showAll(bottles); } } From this program , mix up ink , start with five empty bottles, use random number generator to fill bottles one slot at a time with the colors while keeping 8 slots empty. There is a total of 5×4 =20 slots ,so if we fill 12 slots 8 slots are free.
class WaterSort {
Character top = null;
// create constants for colors
static Character red= new Character('r');
static Character blue = new Character('b');
static Character yellow= new Character('g');
// Bottles declaration
public static void showAll( StackAsMyArrayList bottles[])
{
for (int i = 0; i<=4; i++)
{
System.out.println("Bottle "+ i+ ": " + bottles[i]);
}
}
public static void main(String args[])
{
//part 1
//create the bottle
StackAsMyArrayList bottleONE = new StackAsMyArrayList<Character>();
System.out.println("\nbottleONE:"+ bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.push(red);
bottleONE.push(red);
bottleONE.push(red);
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.push(blue);
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
bottleONE.pop();
bottleONE.pop();
System.out.println("\nbottleONE:"+bottleONE+ " size:" + bottleONE.getStackSize()+" uniform? "+ bottleONE.checkStackUniform());
// Bottles declaration
// Part 2
// Bottles declaration
StackAsMyArrayList bottles[] = new StackAsMyArrayList[5];
//You can do this with a for also
bottles[0] = new StackAsMyArrayList<Character>();
bottles[1] = new StackAsMyArrayList<Character>();
bottles[2] = new StackAsMyArrayList<Character>();
bottles[3] = new StackAsMyArrayList<Character>();
bottles[4] = new StackAsMyArrayList<Character>();
// Fill 3 bottles with specific colors
// need not be uniform colours - just easy to do it with a for
for (int i = 0; i<4; i++)
{
bottles[0].push(red);
bottles[1].push(blue);
bottles[2].push(yellow);
bottles[3].push(yellow);
bottles[4].push(blue);
}
// show all bottles
showAll(bottles);
}
}
From this program , mix up ink , start with five empty bottles, use random number generator to fill bottles one slot at a time with the colors while keeping 8 slots empty. There is a total of 5×4 =20 slots ,so if we fill 12 slots 8 slots are free.

Step by step
Solved in 2 steps with 1 images

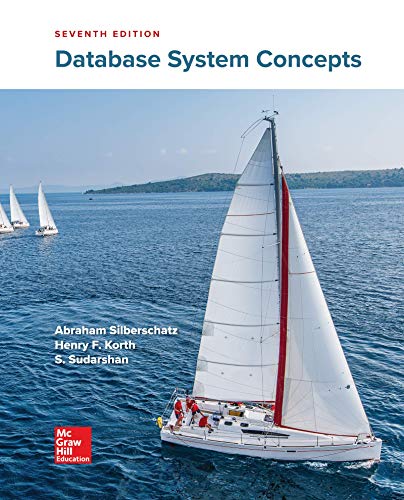
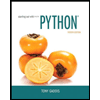
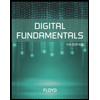
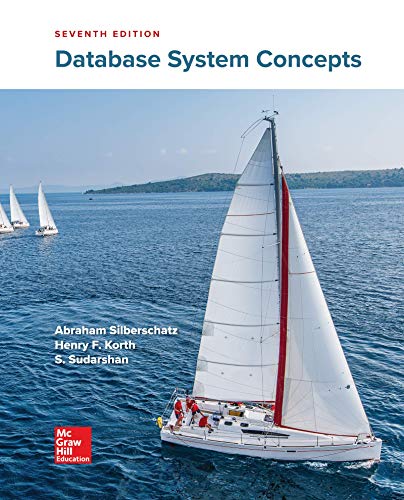
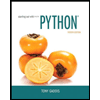
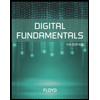
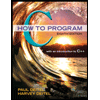
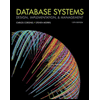
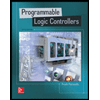