Create a Python file called adopter.py. In this file, write a class called Adopter that matches the following docstring: '''
Create a Python file called adopter.py. In this file, write a class called Adopter that matches the following docstring:
'''
Class -- Adopter
Represents a person that would like to adopt a pet.
Attributes:
name -- The adopter's name, a string.
species_choice -- The species the person would like to adopt e.g. "cat" or "dog"
has_pets -- A boolean, which indicates whether or not the adopter already
has pets.
Method:
...See part B...
'''
Important: make sure the attributes are passed to the constructor in the order shown above. Here's an example showing how an Adopter object will be created:
mr_t = Adopter("Mr. T", "cat", False)
The adopter's name is Mr. T, he would like to adopt a cat, and he does not currently have any pets at home.
Part B
Add a method called is_match to the Adopter class. The method should take a parameter, an instance of a Pet class and return True if the Adopter would be a good match for the Pet and False if not. You do not need to write the Pet class—you can assume someone else has already written it.
Here is all the information you will need:
- The Pet class has two attributes that you will need to use for this part:
- species — a string describing the Pet's species e.g. "cat"
- can_live_with_pets — a boolean which is True if the Pet can live with other pets (meaning it gets along with other animals) and False if not.
- To be considered a match:
- The species of the Pet must match the Adopter's species choice e.g. If the Adopter is looking for a "cat", the Pet must be a "cat". You do not need to worry about string case or spelling of the species e.g. "Cat" would not be a match for "cat".
- If the Adopter has pets, the Pet must be able to live with other pets (assuming the species requirement is met).
- If the Adopter does not have pets, they can be matched with any Pet of the appropriate species.
Here is an example:
fluffy_the_cat = Pet("Fluffy", "cat", True) # Fluffy is a cat that can live with other pets
spot_the_dog = Pet("Spot", "dog", False) # Spot is a dog that cannot live with other pets
mr_t = Adopter("Mr. T", "cat", False)
mr_t.is_match(fluffy_the_cat) # should return True
mr_t.is_match(spot_the_dog) # should return False
Part C
Implement a method in your Adopter class that will make it possible to compare two Adopterobjects using the == operator. Two Adopters should be considered equal if the values of all of their attributes are the same.

Step by step
Solved in 2 steps with 4 images

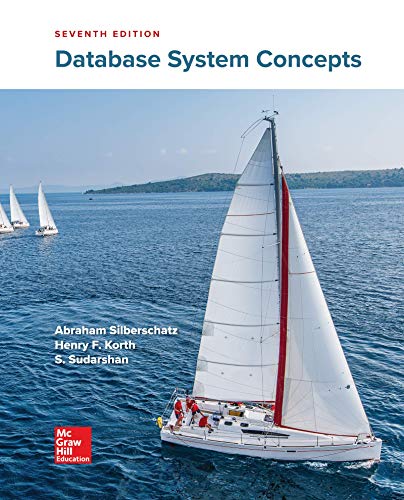
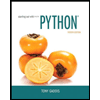
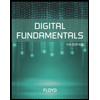
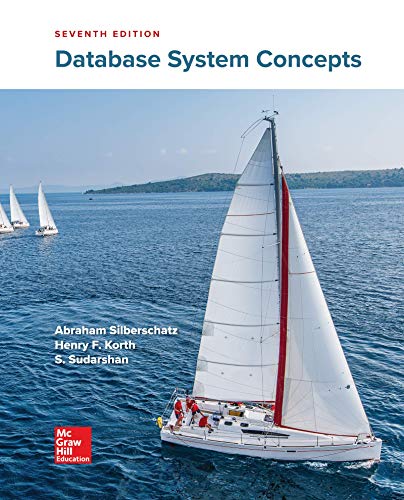
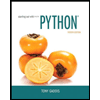
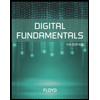
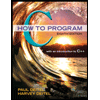
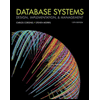
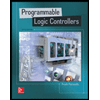