Question 2 - Using functions, design and write a program to calculate students' grades for n students (where n is entered by the user). The program starts by getting the number of students, and all the students names, midterm and final grades. It then computes for each student their average (both exams carry equal weight), and their letter grades ('F'=0..49, 'C'-50..64, 'B'=65..79,'A'= 80..100. At the end, the program prints all the information in a tabular format). Here is a skeleton of your program: const int MAX = 10; // Global constant for the array size // Function to get the information from the user void get_info (int &n, string names [], double mid[], double final []); // Function to calculate the students total mark void calc_averages (int n, double mid[], double final[], double ave[]); // Function to calculate the students letter grades void calc_grades (int n, ave[1, char grades []); // Function to print everything void print_all (int n,string names [], double mid [], double final [], double ave[1, char grades []); int main() { } string names [MAX]; double mid [MAX]; double fin [MAX]; double ave [MAX]; char grades [MAX]; get-info (n, names, mid, final); calc_averages (n, mid, final, ave); calc_grades (n, ave, grades); print_all (n, names, mid, final, ave, grades); A
Question 2 - Using functions, design and write a program to calculate students' grades for n students (where n is entered by the user). The program starts by getting the number of students, and all the students names, midterm and final grades. It then computes for each student their average (both exams carry equal weight), and their letter grades ('F'=0..49, 'C'-50..64, 'B'=65..79,'A'= 80..100. At the end, the program prints all the information in a tabular format). Here is a skeleton of your program: const int MAX = 10; // Global constant for the array size // Function to get the information from the user void get_info (int &n, string names [], double mid[], double final []); // Function to calculate the students total mark void calc_averages (int n, double mid[], double final[], double ave[]); // Function to calculate the students letter grades void calc_grades (int n, ave[1, char grades []); // Function to print everything void print_all (int n,string names [], double mid [], double final [], double ave[1, char grades []); int main() { } string names [MAX]; double mid [MAX]; double fin [MAX]; double ave [MAX]; char grades [MAX]; get-info (n, names, mid, final); calc_averages (n, mid, final, ave); calc_grades (n, ave, grades); print_all (n, names, mid, final, ave, grades); A
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter16: Searching, Sorting And Vector Type
Section: Chapter Questions
Problem 20PE
Related questions
Question
![Question 2
Using functions, design and write a program to calculate students' grades for n students (where n is
entered by the user). The program starts by getting the number of students, and all the students
names, midterm and final grades. It then computes for each student their average (both exams carry
equal weight), and their letter grades ('F'=0..49, 'C'-50..64, 'B'=65..79,'A'= 80..100. At the end, the
program prints all the information in a tabular format).
Here is a skeleton of your program:
const int MAX = 10; // Global constant for the array size
// Function to get the information from the user
void get_info (int &n, string names [], double mid [], double final []);
// Function to calculate the students total mark
void calc_averages (int n, double mid [], double final [], double ave[]);
// Function to calculate the students letter grades
void calc_grades (int n, ave[], char grades[]);
// Function to print everything
void print_all (int n, string names [], double mid[],double final[], double ave[], char grades []);
int main() {
}
string names [MAX];
double mid [MAX];
double fin [MAX];
double ave [MAX];
char grades [MAX];
get-info (n, names, mid, final);
calc averages (n, mid, final, ave);
calc_grades (n, ave, grades);
print_all (n, names, mid, final, ave, grades);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4aa249fd-9146-47ef-8965-21ad8f7ca03d%2F7e6ba728-fd9f-4374-94b5-491b367b0546%2Fgz2jeoh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Question 2
Using functions, design and write a program to calculate students' grades for n students (where n is
entered by the user). The program starts by getting the number of students, and all the students
names, midterm and final grades. It then computes for each student their average (both exams carry
equal weight), and their letter grades ('F'=0..49, 'C'-50..64, 'B'=65..79,'A'= 80..100. At the end, the
program prints all the information in a tabular format).
Here is a skeleton of your program:
const int MAX = 10; // Global constant for the array size
// Function to get the information from the user
void get_info (int &n, string names [], double mid [], double final []);
// Function to calculate the students total mark
void calc_averages (int n, double mid [], double final [], double ave[]);
// Function to calculate the students letter grades
void calc_grades (int n, ave[], char grades[]);
// Function to print everything
void print_all (int n, string names [], double mid[],double final[], double ave[], char grades []);
int main() {
}
string names [MAX];
double mid [MAX];
double fin [MAX];
double ave [MAX];
char grades [MAX];
get-info (n, names, mid, final);
calc averages (n, mid, final, ave);
calc_grades (n, ave, grades);
print_all (n, names, mid, final, ave, grades);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
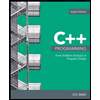
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
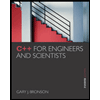
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
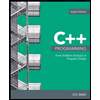
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
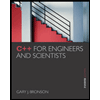
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr