The adjacency matrix that the student computed from the graph above is given by, 0 0 0 1 1 10 1 1 1 1 0 0 0 1 0 1 1 0 Adj = 1 0 0 . 1 0 1 where (Adj)ij, the Element of adjacency matrix at ith row and jth column is: (Adj)ij = 1, if there is a route from city corresponding to jth column to city corresponding to ith row, and: (Adj)ij = 0 otherwise. We give here the correspondence between the indices of the rows and columns and the cities: • First row/column corresponds to city 0, • Second row/column corresponds to city 1, • Third row/column corresponds to city 2, • Fourth row/column corresponds to city 3 and • Fifth row/column corresponds to city 4. For example, we see in the matrix above that: 06 A 23 A 17 2 (Adj) 14 = 1. This means that there is a route from city 4 (corresponding to 4th column) to city 1 (corresponding to 1st row), which corresponds to what we see in the graph. Note: For the following Python questions, you can either fill out the blanks or code them from scratch. You will be able to verify if your code is correct by running "checking code". # This creates the adjacency matrix Adj given above import numpy as np Adj = np.array([[0, 0, 0, 1, 1], [1, 0, 1, 1, 1], [1, 1, 0, 0, 0], [0, 1, 1, 0, 1], [0, 0, 1, 1, 0]]) a) We are interested in knowing the number of connections by water that exist between the five cities. This is the number of connections, or arrows, shown in the graph above. Write a function, called total_routes, that takes the adjacency matrix as input and outputs the number of connections. For example, total_routes (Adj) should return 30 if there are 30 arrows in the above graph. 1 # Input Adjacency matrix, 2 # Output - total count 3 def total_routes (...): 4
The adjacency matrix that the student computed from the graph above is given by, 0 0 0 1 1 10 1 1 1 1 0 0 0 1 0 1 1 0 Adj = 1 0 0 . 1 0 1 where (Adj)ij, the Element of adjacency matrix at ith row and jth column is: (Adj)ij = 1, if there is a route from city corresponding to jth column to city corresponding to ith row, and: (Adj)ij = 0 otherwise. We give here the correspondence between the indices of the rows and columns and the cities: • First row/column corresponds to city 0, • Second row/column corresponds to city 1, • Third row/column corresponds to city 2, • Fourth row/column corresponds to city 3 and • Fifth row/column corresponds to city 4. For example, we see in the matrix above that: 06 A 23 A 17 2 (Adj) 14 = 1. This means that there is a route from city 4 (corresponding to 4th column) to city 1 (corresponding to 1st row), which corresponds to what we see in the graph. Note: For the following Python questions, you can either fill out the blanks or code them from scratch. You will be able to verify if your code is correct by running "checking code". # This creates the adjacency matrix Adj given above import numpy as np Adj = np.array([[0, 0, 0, 1, 1], [1, 0, 1, 1, 1], [1, 1, 0, 0, 0], [0, 1, 1, 0, 1], [0, 0, 1, 1, 0]]) a) We are interested in knowing the number of connections by water that exist between the five cities. This is the number of connections, or arrows, shown in the graph above. Write a function, called total_routes, that takes the adjacency matrix as input and outputs the number of connections. For example, total_routes (Adj) should return 30 if there are 30 arrows in the above graph. 1 # Input Adjacency matrix, 2 # Output - total count 3 def total_routes (...): 4
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python Help(I need code for Part A)

Transcribed Image Text:A student is interested in traveling by water between 5 cities that are called 0, 1, 2, 3 and 4 and that are shown in the graph
below. If there is a connection between two given cities by water, then the two cities are connected by an arrow in the graph.
For example, there is a connection by water going from city 0 to city 1.
The student created the adjacency matrix of the graph, which is a matrix of Os and 1s. The definition of adjacency matrix
was seen in class and is recalled below.
The student is having trouble answering some questions related to the travel as he/she is not able to make inferences from
the adjacency matrix that was created successfully.
Let's provide some help.
4
m
![The adjacency matrix that the student computed from the graph above is given by,
0 1 1
1
1
0
0
1
1 0
Adj
0
0
0
1
1
0 1
where (Adj)ij, the Element of adjacency matrix at ith row and jth column is:
(Adj)ij
1, if there is a route from city corresponding to jth column to city corresponding to ith row, and:
• (Adj)ij = 0 otherwise.
=
We give here the correspondence between the indices of the rows and columns and the cities:
• First row/column corresponds to city 0,
• Second row/column corresponds to city 1,
• Third row/column corresponds to city 2,
• Fourth row/column corresponds to city 3 and
• Fifth row/column corresponds to city 4.
For example, we see in the matrix above that:
This means that there is a route from city 4 (corresponding to 4th column) to city 1 (corresponding to 1st row), which
corresponds to what we see in the graph.
(Adj) 14
Note: For the following Python questions, you can either fill out the blanks or code them from scratch. You will be able to
verify if your code is correct by running "checking code".
# Input - Adjacency matrix,
= 1.
# This creates the adjacency matrix Adj given above
import numpy as np
Adj = np.array([[0, 0, 0, 1, 1], [1, 0, 1, 1, 1], [1, 1, 0, 0, 0], [0, 1, 1, 0, 1], [0, 0, 1, 1, 0]])
1
2 # Output - total count
3 def total_routes (...):
4
5
6
7
8
90
06 A 23 A 172
a) We are interested in knowing the number of connections by water that exist between the five cities. This is the number of
connections, or arrows, shown in the graph above. Write a function, called total_routes, that takes the adjacency matrix as
input and outputs the number of connections. For example, total_routes (Adj) should return 30 if there are 30 arrows in the
above graph.
# Add your code
return...
Add Code Cell Add Markdown Cell](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F584ffa08-94ad-4eb4-a87d-fd258db9f802%2Fb28db96b-c54d-407a-994d-c3538783f5b4%2F76azka_processed.png&w=3840&q=75)
Transcribed Image Text:The adjacency matrix that the student computed from the graph above is given by,
0 1 1
1
1
0
0
1
1 0
Adj
0
0
0
1
1
0 1
where (Adj)ij, the Element of adjacency matrix at ith row and jth column is:
(Adj)ij
1, if there is a route from city corresponding to jth column to city corresponding to ith row, and:
• (Adj)ij = 0 otherwise.
=
We give here the correspondence between the indices of the rows and columns and the cities:
• First row/column corresponds to city 0,
• Second row/column corresponds to city 1,
• Third row/column corresponds to city 2,
• Fourth row/column corresponds to city 3 and
• Fifth row/column corresponds to city 4.
For example, we see in the matrix above that:
This means that there is a route from city 4 (corresponding to 4th column) to city 1 (corresponding to 1st row), which
corresponds to what we see in the graph.
(Adj) 14
Note: For the following Python questions, you can either fill out the blanks or code them from scratch. You will be able to
verify if your code is correct by running "checking code".
# Input - Adjacency matrix,
= 1.
# This creates the adjacency matrix Adj given above
import numpy as np
Adj = np.array([[0, 0, 0, 1, 1], [1, 0, 1, 1, 1], [1, 1, 0, 0, 0], [0, 1, 1, 0, 1], [0, 0, 1, 1, 0]])
1
2 # Output - total count
3 def total_routes (...):
4
5
6
7
8
90
06 A 23 A 172
a) We are interested in knowing the number of connections by water that exist between the five cities. This is the number of
connections, or arrows, shown in the graph above. Write a function, called total_routes, that takes the adjacency matrix as
input and outputs the number of connections. For example, total_routes (Adj) should return 30 if there are 30 arrows in the
above graph.
# Add your code
return...
Add Code Cell Add Markdown Cell
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
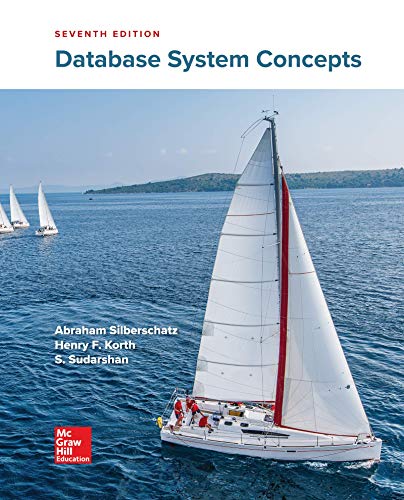
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
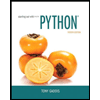
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
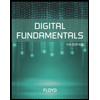
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
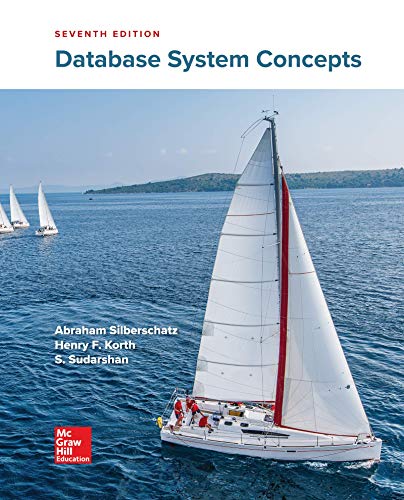
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
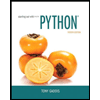
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
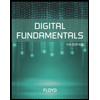
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
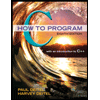
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
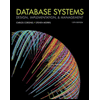
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
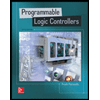
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education