Using Java. Implement a deque, except base your implementation on an array. The constructor for the class should accept an integer parameter for the capacity of the deque and create an array of that size. Use a graphical user interface based on an array of text fields similar to what is shown in the image attached. Test your deque class by constructing a deque with capacity 10. Below also is an unfinished code for the challenge. ################################# Array Based Deque ################################# public class ArrayBaseDeque { private String [ ] q; // Holds queue elements private int front; // Next item to be removed private int rear; // Next slot to fill private int size; // Number of items in queue private int count = 0; /** Constructor. @param capacity The capacity of the queue. */ ArrayBaseDeque(int capacity) { q = new String[capacity]; front = 0; rear = 0; size = 0; } /** The capacity method returns the length of the array used to implement the queue. @return The capacity of the queue. */ public void addFront(String e){ if(count == q.length) throw new RuntimeException("The deque is full."); // add to front q[front] = e; front --; if (front == -1) front = q.length-1; count ++; } public void addRear(String e){ if(count == q.length) throw new RuntimeException("The deque is full."); // add to rear q[rear] = e; rear --; if(rear == -1) rear = q.length-1; count ++; } public String removeFront(){ if(count == 0) throw new RuntimeException("Queue is empty."); front ++; if(front == q.length) front = 0; String e = q[front]; q[front] = null; count --; return e; } public String removeRear(){ if(count == 0) throw new RuntimeException("Queue is empty."); rear ++; if(rear == q.length) rear = 0; String e = q[rear]; q[rear] = null; count --; return e; } } ################################# Array Based Deque Demo ################################# import java.awt.*; import javax.swing.*; import java.awt.event.*; public class ArrayBaseDequeDemo extends JFrame { private ArrayBaseDeque deque = new ArrayBaseDeque(10); private JTextField []dViewTextField = new JTextField [10]; private JTextField cmdTextField = new JTextField (10); private JLabel frontLabel = new JLabel(); private JLabel rearLabel = new JLabel(); private JLabel resultLabel = new JLabel(); public static void main(String [] args) { // Complete the code ....... } }
Using Java. Implement a deque, except base your implementation on an array. The constructor for the class should accept an integer parameter for the capacity of the deque and create an array of that size.
Use a graphical user interface based on an array of text fields similar to what is shown in the image attached. Test your deque class by constructing a deque with capacity 10.
Below also is an unfinished code for the challenge.
#################################
Array Based Deque
#################################
public class ArrayBaseDeque
{
private String [ ] q; // Holds queue elements
private int front; // Next item to be removed
private int rear; // Next slot to fill
private int size; // Number of items in queue
private int count = 0;
/**
Constructor.
@param capacity The capacity of the queue.
*/
ArrayBaseDeque(int capacity)
{
q = new String[capacity];
front = 0;
rear = 0;
size = 0;
}
/**
The capacity method returns the length of
the array used to implement the queue.
@return The capacity of the queue.
*/
public void addFront(String e){
if(count == q.length)
throw new RuntimeException("The deque is full.");
// add to front
q[front] = e;
front --;
if (front == -1)
front = q.length-1;
count ++;
}
public void addRear(String e){
if(count == q.length)
throw new RuntimeException("The deque is full.");
// add to rear
q[rear] = e;
rear --;
if(rear == -1)
rear = q.length-1;
count ++;
}
public String removeFront(){
if(count == 0)
throw new RuntimeException("Queue is empty.");
front ++;
if(front == q.length)
front = 0;
String e = q[front];
q[front] = null;
count --;
return e;
}
public String removeRear(){
if(count == 0)
throw new RuntimeException("Queue is empty.");
rear ++;
if(rear == q.length)
rear = 0;
String e = q[rear];
q[rear] = null;
count --;
return e;
}
}
#################################
Array Based Deque Demo
#################################
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class ArrayBaseDequeDemo extends JFrame
{
private ArrayBaseDeque deque = new ArrayBaseDeque(10);
private JTextField []dViewTextField = new JTextField [10];
private JTextField cmdTextField = new JTextField (10);
private JLabel frontLabel = new JLabel();
private JLabel rearLabel = new JLabel();
private JLabel resultLabel = new JLabel();
public static void main(String [] args)
{
// Complete the code .......
}
}
![Grading - NetBeans IDE 8.2
<default conf... T
Qv Search (% +1)
Projects
Files
Services
Start Page
ArrayBasedDequeDemo.java
JavaFXDoubleEndedQueueDemo.java x
A ArrayBasedDeque.java X
Grading
Source Packages
Source
History
1 e import java.awt.*;
import javax. swing.*;
import java. awt.event.*;
<default package>
Av...
2
ArrayBased Deque.java
* ArrayBased DequeDemo.java
JavaFXDoubleEndedQueueDemo.java
addfront element
addrear element
removefront
3
4
0 1 2 3 4 5 6 7 8 9
/**
Test Packages
removerear
Programming Challenge 21-2.
Array-based deaue Demo.
*/
Deque Command:
Libraries
isempty
7
size
Test Libraries
10
public class ArrayBasedDequeDemo extends JFrame
{
11
12
13
= new ArrayBasedDeque (10);
private ArrayBasedDeque deque
private JTextField [] dViewTextField = new JTextField [10];
private JTextField cmdTextField = new JTextField(10);
17
private JLabel frontLabel = new JLabel();
private JLabel rearLabel = new JLabel();
private JLabel resultLabel = new JLabel();
HA
HA
Navigator
21
Members
<empty>
22
23 E
ArrayBasedDequeDemo : JFrame
ArrayBased DequeDemo()
main(String[] args)
cmdTextField : JTextField
dViewTextField : JTextField[]
/**
This class listens to commands typed in at the cmdTextField
and passes them to the deque. It then displays the result
of executing that command on the deaue in the view text fields.
24
25
26
27
*/
28
private class CmdTextListener implements ActionListener
deque : ArrayBasedDeque
frontLabel : JLabel
Output - Grading (run)
rearLabel : JLabel
run:
resultLabel : JLabel
CmdTextListener :: ActionListener
actionPerformed(ActionEvent evt)
displayDeque()
HelpDialog : JDialog
HelpDialog(String[] cmds)
Grading (run)
running...
1:1
INS
........
<>](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8981e183-e99b-4707-a5da-051f46ea9291%2Fa432d1e9-6785-4821-974c-d659d9a12387%2Fy9ix7y3_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

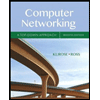
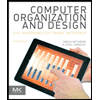
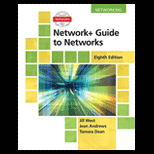
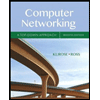
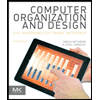
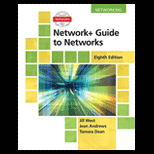
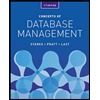
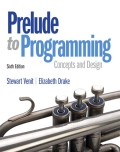
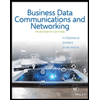