You are writing a program to track apples grown over the last 8 years at the NVCC Apple Farm. Create a class called Apples that contains the following: • a data member for an array to hold apple data • a constructor that accepts a parameter for an array that stores the apples grown each year • a method that calculates the total apples grown over 8 years • a method that calculates the average apples grown over 8 years a method that gets the highest apples grown over 8 years • a method that gets the lowest apples grown over 8 years • method that returns the element number Create a program called ApplesDemo that references the Apples class that contains the following: • an array to store the number of apples grown each year • an array to store the 8 years • a for loop that prompts the user to enter the number of apples grown for each year • create an object that represents the apples stored within the array • for the object created, run the method that totals the apples grown over the 8 years and displays the result • for the object created, run the method that averages the apples grown over the 8 years and displays the result for the object created, run the method that gets the highest number of apples grown over the 8 year period and display the result for the object created, run the method that gets the lowest number of apples grown over the 8 year period and display the result Use printf in order to control the placement of the comma in the results and to show 1 decimal place as shown in the results below. Other reminders: Use camelCase correctly Indent correctly Comment at the top of each program and within each program Use the test data given for testing. Here is how the program will run. Test data input is italicized and bolded.
You are writing a program to track apples grown over the last 8 years at the NVCC Apple Farm. Create a class called Apples that contains the following: • a data member for an array to hold apple data • a constructor that accepts a parameter for an array that stores the apples grown each year • a method that calculates the total apples grown over 8 years • a method that calculates the average apples grown over 8 years a method that gets the highest apples grown over 8 years • a method that gets the lowest apples grown over 8 years • method that returns the element number Create a program called ApplesDemo that references the Apples class that contains the following: • an array to store the number of apples grown each year • an array to store the 8 years • a for loop that prompts the user to enter the number of apples grown for each year • create an object that represents the apples stored within the array • for the object created, run the method that totals the apples grown over the 8 years and displays the result • for the object created, run the method that averages the apples grown over the 8 years and displays the result for the object created, run the method that gets the highest number of apples grown over the 8 year period and display the result for the object created, run the method that gets the lowest number of apples grown over the 8 year period and display the result Use printf in order to control the placement of the comma in the results and to show 1 decimal place as shown in the results below. Other reminders: Use camelCase correctly Indent correctly Comment at the top of each program and within each program Use the test data given for testing. Here is how the program will run. Test data input is italicized and bolded.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
using jgrasp please write the following JAVA code with the following directions, there's an example for your reference as well
The total number of apples grown in the last 8 years is 68,700.0
The average number of apples grown in the last
8 years is 8,587.5
The year with the largest number of apples grown is 2019 with 11, 000.0 apples
grown.
The year with the smallest number of apples grown in 2015 with 5,300.0 apples
grown.

Transcribed Image Text:You are writing a program to track apples grown over the last 8 years at the NVCC Apple Farm.
Create a class called Apples that contains the following:
a data member for an array to hold apple data
• a constructor that accepts a parameter for an array that stores the apples grown each year
• a method that calculates the total apples grown over 8 years
• a method that calculates the average apples grown over 8 years
• a method that gets the highest apples grown over 8 years
a method that gets the lowest apples grown over 8 years
method that returns the element number
Create a program called ApplesDemo that references the Apples class that contains the following:
an array to store the number of apples grown each year
• an array to store the 8 years
• a for loop that prompts the user to enter the number of apples grown for each year
• create an object that represents the apples stored within the array
• for the object created, run the method that totals the apples grown over the 8 years and displays the
result
• for the object created, run the method that averages the apples grown over the 8 years and displays the
result
for the object created, run the method that gets the highest number of apples grown over the 8 year
period and display the result
for the object created, run the method that gets the lowest number of apples grown over the 8 year
period and display the result
Use printf in order to control the placement of the comma in the results and to show 1 decimal place as
shown in the results below.
Other reminders:
Use camelCase correctly
Indent correctly
Comment at the top of each program and within each program
Use the test data given for testing.
Here is how the program will run. Test data input is italicized and bolded.

Transcribed Image Text:Here is how the program will run. Test data input is italicized and bolded.
Please enter the number of apples grown at the NVCC Apple Farm in 2013
5500
Please enter the number of apples grown at the NVCC Apple Farm in 2014
7500
Please enter the number of apples grown at the NVCC Apple Farm in 2015
5300
Please enter the number of apples grown at the NVCC Apple Farm in 2016
8800
Please enter the number of apples grown at the NVCC Apple Farm in 2017
9200
Please enter the number of apples grown at the NVCC Apple Farm in 2018
10500
Please enter the number of apples grown at the NVCC Apple Farm in 2019
11000
Please enter the number of apples grown at the NVCC Apple Farm in 2020
10900
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
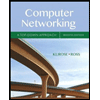
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
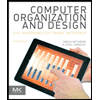
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
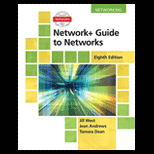
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
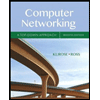
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
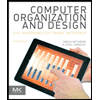
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
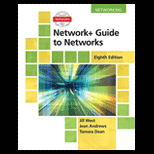
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
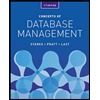
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
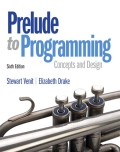
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
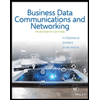
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY