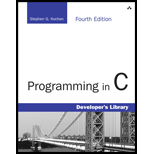
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11, Problem 8E
Program Plan Intro
Program Plan:
- Include the necessary header files into program.
- Define the function named “bitSize()” which is used to fetch the size of the value.
- Declare the variables in type of unsigned int and int.
- Using “while” loop, check the value of “size”.
- If the value greater than “0”, right shift operation is performed and increments the “temp” value.
- Return the value of “temp-1”.
- Define the function named “no_of_bits()” which is used to calculate the minimum number of bits needed to represent the value.
- Declare and initialize the variables.
- Using “while” loop, check the value and left shift the value of “temp” and increment the “counter” value.
- Return the size which is reduced from counter.
- Define the function named “bitpat_get()” which is used to extract the “n” bits from source starting at “start” argument.
- Declare the variables.
- Call the “bitSize()” method and store the size into variable “size”.
- Initialize the value of “c” in type of integer.
- Using “if…else” condition, calculate the offset and temporary value.
- Return the temporary value to function.
- Define the function named “bitpat_set()” which is used to set the bit size within the source to value which is starting at “*start”.
- Declare the variables.
- Call the “no_of_bits()” method which passes “*start” as argument.
- Call the other appropriate methods and store in the variable.
- Using left shift operator to calculate the value of “val”.
- Print the appropriate result on screen.
- Define the main method.
- Declare the variables in type of unsigned integer.
- Prompt and get the values.
- Call the “bitpat_set()” method and print the result on screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Start by writing a function, plot_grades (filename, first_name, last_name),
which plots the grades for the specified student. This will be very similar in nature to
what you did in Assignment 5.2 but will use the csv library instead of split ().
a. The function should find the specified student in the file associated with
filename.
b. It should use plotter to plot all of the graded items for that student.
c. Manually test your function using the full grades_ XXX.csv files.
Let A and B two text files, containing some binary lines between of length 1...N. Where N is the line with the maximum number of characters, 1 the minimum number of characters in each line.
Design a Python3 function to compare each line of the files, character by character, and if there is a match of the characters, create a variable representing the similarity between the lines of each file, and return that similarity variable measures the similarity between two files.
Write a recursive function, displayFiles, that expects a pathname as an argument. The path name can be either the name of a file or the name of a directory. If the pathname refers to a file, its filepath is displayed, followed by its contents, like so:
File name: file_path Lorem ipsum dolor sit amet, consectetur adipiscing elit...
Otherwise, if the pathname refers to a directory, the function is applied to each name in the directory, like so:
Directory name: directory_path File name: file_path1 Lorem ipsum dolor sit amet... File name: file_path2 Lorem ipsum dolor sit amet... ...
Chapter 11 Solutions
Programming in C
Ch. 11 - Type in and run the four programs presented in...Ch. 11 - Write a program that determines whether your...Ch. 11 - Prob. 3ECh. 11 - Using the result obtained in exercise 3, modify...Ch. 11 - Write a function called b that takes two...Ch. 11 - Write a function called b that looks for the...Ch. 11 - Write a function called b to extract a specified...Ch. 11 - Prob. 8E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a recursive function, displayFiles, that expects a pathname as an argument. The path name can be either the name of a file or the name of a directory. If the pathname refers to a file, its filepath is displayed, followed by its contents, like so: File name: file_path Lorem ipsum dolor sit amet, consectetur adipiscing elit... Otherwise, if the pathname refers to a directory, the function is applied to each name in the directory, like so: Directory name: directory_path File name: file_path1 Lorem ipsum dolor sit amet... File name: file_path2 Lorem ipsum dolor sit amet... ... # Put your code here import os #module used to interact with operating system def displayFiles(pathname): #recursive function that takes a pathname as argument if (os.path.isdir(pathname)): #checks if specified path (argument) is an existing directory #for item in os.listdir(pathname): for content in os.listdir(pathname): #gets the list of all files and directories in the directory and…arrow_forwardThank youarrow_forwardThank youarrow_forward
- Thank youarrow_forwardWrite a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardThis problem needs to be solved in python. Write a function read_movies_data(f) that takes in a movies file and returns a pandas DataFrame, where the index is the movie id and the columns are "title", "year", and "genre" (in that order). movies_df = read_movies_data("moviesSample.txt") assert movies_df.shape == (9, 3)assert movies_df.iloc[2, 2] == "Comedy"assert movies_df.loc[6, "title"] == "Heat"assert all(movies_df.columns == ["title", "year", "genre"])assert list(movies_df.index) == [1, 2, 3, 4, 5, 6, 8, 9, 10] NOTE: Make sure all of the asserts work The moviesSample.txt looks like this: 1|Toy Story|1995|Adventure2|Jumanji|1995|Adventure8|Tom and Huck|1995|Adventure3|Grumpier Old Men|1995|Comedy4|Waiting to Exhale|1995|Comedy5|Father of the Bride Part II|1995|Comedy6|Heat|1995|Action9|Sudden Death|1995|Action10|GoldenEye|1995|Action NOTE: Try to sent screenshots of the code working.arrow_forward
- Write a C/C++ program that calculates the least squares line for two sets of values. The program consists of several functions which are described below. int ReadFile (int *, int *); Write a function that reads the contents of a text file (Data1.txt) into two separate integer arrays. The function receives two integer pointers to arrays as parameters. The contents of the text file that is attached is structured as follows: Each line in the file contains two values that are separated by a comma. The values on the left of the comma, are stored in one array and the values on the right side is stored in the other array. The number of lines that are read from the file and stored in the arrays are returned to the calling statement. Tip: a single fscanf can read into multiple variables. Ex. the statement fscanf(fp, "%s %s\n", name,surname) will read the line John Doe and save the name and surname separately. (Note the space between %s and %s indicates that there is a space in the data between…arrow_forwardWrite a C/C++ program that calculates the least squares line for two sets of values. The program consists of several functions which are described below. int ReadFile (int *, int *); Write a function that reads the contents of a text file (Data1.txt) into two separate integer arrays. The function receives two integer pointers to arrays as parameters. The contents of the text file that is attached is structured as follows: Each line in the file contains two values that are separated by a comma. The values on the left of the comma, are stored in one array and the values on the right side is stored in the other array. The number of lines that are read from the file and stored in the arrays are returned to the calling statement. Tip: a single fscanf can read into multiple variables. Ex. the statement fscanf(fp, "%s %s\n", name,surname) will read the line John Doe and save the name and surname separately. (Note the space between %s and %s indicates that there is a space in the data between…arrow_forwardWrite a C/C++ program that calculates the least squares line for two sets of values. The program consists of several functions which are described below. int ReadFile (int *, int *); Write a function that reads the contents of a text file (Data1.txt) into two separate integer arrays. The function receives two integer pointers to arrays as parameters. The contents of the text file that is attached is structured as follows: Each line in the file contains two values that are separated by a comma. The values on the left of the comma, are stored in one array and the values on the right side is stored in the other array. The number of lines that are read from the file and stored in the arrays are returned to the calling statement. Tip: a single fscanf can read into multiple variables. Ex. the statement fscanf(fp, "%s %s\n", name,surname) will read the line John Doe and save the name and surname separately. (Note the space between %s and %s indicates that there is a space in the data between…arrow_forward
- Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents of movies.csv…arrow_forwardWrite a C/C++ program that calculates the least squares line for two sets of values. The program consists of several functions which are described below. int ReadFile (int *, int *); Write a function that reads the contents of a text file (Data1.txt) into two separate integer arrays. The function receives two integer pointers to arrays as parameters. The contents of the text file that is attached is structured as follows: Each line in the file contains two values that are separated by a comma. The values on the left of the comma, are stored in one array and the values on the right side is stored in the other array. The number of lines that are read from the file and stored in the arrays are returned to the calling statement. Tip: a single fscanf can read into multiple variables. Ex. the statement fscanf(fp, "%s %s\n", name,surname) will read the line John Doe and save the name and surname separately. (Note the space between %s and %s indicates that there is a space in the data between…arrow_forwardWrite a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents of movies.csv…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
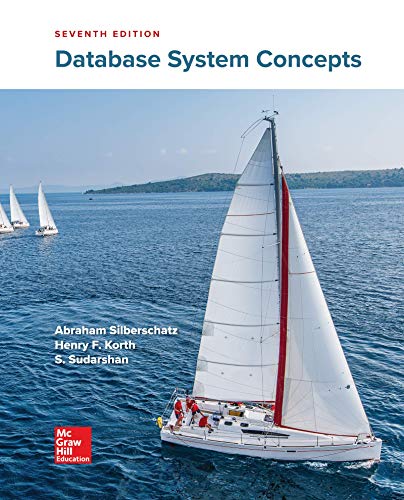
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
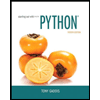
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
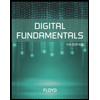
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
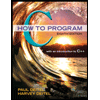
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
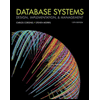
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
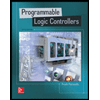
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education