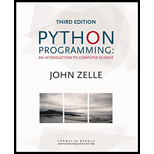
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 6PE
Program Plan Intro
Printing digits of a number
- Define a class “BaseConversion”.
- Define “init” function.
- Declaring and initializing instance variables.
- Define a function “makeList()”.
- Extract the digits.
- Append the digit.
- Divide the value of “num” by “base”.
- If the condition “self.num < self.base” is true
- Set the value of “digit”.
- Set the value of “num” as 0.
- Append the value of “digit”.
- If the condition is not true.
- Call the function “makeList()”.
- Define a function “getDigits(self)”.
- Call a function “reverse()”.
- Define the array of digit strings.
- Define an array “newlist”.
- Iterate a “for” loop.
- Set the value of “num” with array values.
- Append the value of num.
- Creating a string “str1”.
- Define “init” function.
- Call the function “BaseConversion()”.
- Call the function “getDigits()”.
- Printing the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a program that asks a number “N” from user. Write a function that takes this number “N”, then returns the sum of numbers starting from N to 1. Use this function in your program and show the results. Be careful, your function should be recursive!
I need code on C programming
Write a recursive function that returns true if the digits of a positive integer are in increasing order; otherwise, the function returns false. Also, write a program to test your function.
Computer scientists and mathematicians often use numbering systems other than base 10. Write a program that allows a user to enter a number and a
base and then prints out the digits of the number in the new base. Use a recursive function baseConversion (num, base) to print the digits.
Hint: Consider base 10. To get the rightmost digit of a base 10 number, simply look at the remainder after dividing by 10. For example, 153 % 10
is 3. To get the remaining digits, you repeat the process on 15, which is just 153 // 10. This same process works for any base. The only problem
is that we get the digits in reverse order (right to left).
The base case for the recursion occurs when num is less than base and the output is simply num. In the general case, the function (recursively)
prints the digits of num // base and then prints num % base. You should put a space between successive outputs, since bases greater than 10 will
print out with multi-character "digits." For example, baseConversion(1234,…
Chapter 13 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 13 - Prob. 1TFCh. 13 - Prob. 2TFCh. 13 - Prob. 3TFCh. 13 - Prob. 4TFCh. 13 - Prob. 5TFCh. 13 - Prob. 6TFCh. 13 - Prob. 7TFCh. 13 - Prob. 8TFCh. 13 - Prob. 9TFCh. 13 - Prob. 10TF
Ch. 13 - Prob. 1MCCh. 13 - Prob. 2MCCh. 13 - Prob. 3MCCh. 13 - Prob. 4MCCh. 13 - Prob. 5MCCh. 13 - Prob. 6MCCh. 13 - Prob. 7MCCh. 13 - Prob. 8MCCh. 13 - Prob. 9MCCh. 13 - Prob. 10MCCh. 13 - Prob. 1DCh. 13 - Prob. 2DCh. 13 - Prob. 3DCh. 13 - Prob. 4DCh. 13 - Prob. 5DCh. 13 - Prob. 1PECh. 13 - Prob. 2PECh. 13 - Prob. 3PECh. 13 - Prob. 4PECh. 13 - Prob. 5PECh. 13 - Prob. 6PECh. 13 - Prob. 7PE
Knowledge Booster
Similar questions
- Write a recursive function, sumDigits, that takes an integer as a parameter and returns the sum of the digits of the integer. Also, write a program to test your function.arrow_forwardConsider a network of streets laid out in a rectangular grid, In a northeast path from one point in the grid to another, one may walk only to the north (up) and to the east (right). Write a program that must use a recursive function to count the number of northeast paths from one point to another in a rectangular grid. Your program should prompt the user to input the numbers of points to the north and to east respectively, and then output the total number of paths. Notes: 1. Here is a file (timer.h download and timer.cpp download) which should be included in your program to measure time in Window or Unix (includes Mac OS and Linux) systems (use start() for the beginning of the algorithm, stop() for the ending, and show() for printing). 2. The computing times of this algorithm is very high, and the number of paths may be overflow, don't try input numbers even over 16. 3. Name your recursive function prototype as calcPath(int north, int east) to ease grading. 4. Paste your outputarrow_forwardWrite a recursive function that returns a value of 1 if its string argument is apalindrome and zero otherwise.* Note that for the function parameters, you need to accommodate for the shrinkingstring, so that the string shrinks both from beginning and end after each recursive call.** Think about the simple cases or base cases. The 2 base cases have conditions thatwould return 0 and return 1 independently.For the palindrome, create a driver program. First ask the user to enter anystring through the keyboard. Then remove all the spaces and punctuations from thestring. Also, remove any letter capitalization from the string. Finally, pass the string tothe palindrome function through a function call.arrow_forward
- write a recursive function named choose (int n,int k) that will compute and return the value of the binomial coefficient. write it as a C++ function on the computer.arrow_forwardWrite a recursive function that takes as a parameter a nonnegative integer and generates the following pattern of stars. If the nonnegative integer is 4, then the pattern generated is:********************Also, write a program that prompts the user to enter the number of lines in the pattern and uses the recursive function to generate the pattern. For example, specifying 4 as the number of lines generates the above pattern.arrow_forwardWrite a recursive function to print all the permutations of a string. For example, for the string abc, the printout is:abcacbbacbcacabcba(Hint: Define the following two functions. The second function is a helper function.def displayPermuation(s):def displayPermuationHelper(s1, s2): The first function simply invokes displayPermuation(" ", s). The secondfunction uses a loop to move a character from s2 to s1 and recursively invokes t with a new s1 and s2. The base case is that s2 is empty and prints s1 to the console.)Write a test program that prompts the user to enter a string and displays all its permutations.arrow_forward
- Write a recursive function that takes a positive integer and returns the factorial of that integer. Attention, the function must be recursive, and its name must be "fatorial".arrow_forwardWrite a program that allows a user to enter non-negative int number. The recursive function is to be named sumSquares that returns the sum of the squares of the numbers from 0 to number.Do not use any global variables.arrow_forwardWrite a recursive function named sumSquares that returns the sum of the squares of the numbers from 0 to num, in which num is a nonnegative int variable. Do not use global variables; use the appropriate parameters. Also write a program to test your function.arrow_forward
- A palindrome is a sentence that contains the same sequence of letters reading it either forwards or backwards. A classic example is '1\.ble was I, ereI saw Elba." Write a recursive function that detects whether a string is apalindrome. The basic idea is to check that the first and last letters of thestring are the same letter; if they are, then the entire string is a palindromeif everything between those letters is a palindrome.There are a couple of special cases to check for. If either the first orlast character of the string is not a letter, you can check to see if the restof the string is a palindrome with that character removed. Also, when youcompare letters, make sure that you do it in a case-insensitive way.Use your function in a program that prompts a user for a phrase andthen tells whether or not it is a palindrome. Here's another classic fortesting: '1\. man, a plan, a canal, Panama!"arrow_forwardFibonacci numbers are a sequence of integers, starting with 1, where the value of each number is the sum of the two previous numbers, e.g. 1, 1, 2, 3, 5, 8, etc. Write a function called fibonacci that takes a parameter, n, which contains an integer value, and have it return the nth Fibonacci number. (There are two ways to do this: one with recursion, and one without.)arrow_forwardWrite a recursive function that finds the number of occurrences of a specified letter in a string using the following function header. def count(s, a):For example, count("Welcome", 'e') returns 2. Write a test program thatprompts the user to enter a string and a character, and displays the number of occurrences for the character in the string.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
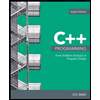
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning