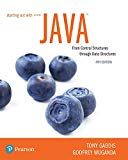
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17, Problem 6PC
Program Plan Intro
Generic class
Program plan:
- Import the required package.
- Create the class “MyList” with a type parameter “T” that accepts any type that implements “Comparable” interface.
- Create “ArrayList” as a field of class “MyList” with type parameter “T”.
- Define the constructor.
- Define the public method “add()” to accept the parameter of type “T”,
- Store the value in “ArrayList” using the reference variable “list”.
- Define the method “largest()”,
- Call the method “get()” to store the initial value of “ArrayList” in the variable “val”.
- Iterate the elements of “ArrayList” using “for” loop.
- Use standard method “compareTo()” for standard comparison to find largest value..
- Return the largest value using the keyword “return”.
- Define the method “smallest()”,
- Call the method “get()” to store the initial value of “ArrayList” in the variable “val”.
- Iterate using “for” loop to find the smallest value in the “ArrayList”.
- Use standard method “compareTo()” for standard comparison to find smallest value.
- Return the smallest value using the keyword “return”.
- Create the class “Main”,
- Define the method “main()”,
- Create the object of a class “MyList” as a type of “Integer”.
- Call the “add()” method using the object of type “Integer” to fill the values in the “ArrayList” of “Integer”.
- Call the method “largest()” to print the largest value of Integer “ArrayList”.
- Call the method “smallest()” to print the smallest value of Integer “ArrayList”.
- Define the method “main()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
public class Point {
Create two variables:
1. Generic Variable:
Variable Name: data
2. Generic Point:
Varibale Name: next
10
11
12
13
/*
14
Constructor that takes in
two parameters (see above comment)
*/
15
16
17
18
19
20
/*
21
Setters and getters
22
*/
23
24
wwwm
25
26
/*
27
tostring: output should be in the format:
28
(data)--> (next)
29
Example:
30
Point p1,p2;
31
p1.data = 4;
32
p1.next = p2;
33
p1.toString () would be: (4) -->(p2)
34
35
36
Computer Engineering lab
Assignment 3:- Apex (Salesforce) - Create an apex class - In the apex class we have to create 1 method. - Method return type is void & the argument is null. - Method : - Map<String,String> static 4 values---- Put the value at the time of initialization---- Put the value using the map method PUT.
Make Album in c++
You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features:
Attributes:
Album Title
Artist Name
Array of song names (Track Listing)
Array of track lengths (decide on a unit)
At most there can be 20 songs on an album.
Behaviors:
Default and at least one argumented constructor
Appropriate getters for attributes
bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is.
getAlbumLength() - method dat returns total length of album matching watever units / type you decide.
getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here.
string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album.
The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)...
Well formatted print()…
Chapter 17 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - When ArrayList is used as a non-generic class, why...Ch. 17.1 - Suppose we use the following statement to...Ch. 17.1 - Assume we have used the statement shown in...Ch. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Prob. 17.7CPCh. 17.2 - Prob. 17.8CPCh. 17.3 - Prob. 17.9CPCh. 17.3 - Prob. 17.10CP
Ch. 17.3 - Prob. 17.11CPCh. 17.3 - Prob. 17.12CPCh. 17.3 - Prob. 17.13CPCh. 17.3 - Prob. 17.14CPCh. 17.4 - Prob. 17.15CPCh. 17.5 - Prob. 17.16CPCh. 17.5 - Prob. 17.17CPCh. 17.6 - Prob. 17.18CPCh. 17.6 - Prob. 17.19CPCh. 17.6 - Prob. 17.20CPCh. 17.8 - Prob. 17.21CPCh. 17.8 - Prob. 17.22CPCh. 17.9 - Prob. 17.23CPCh. 17.9 - During the process of erasure, when the compiler...Ch. 17.9 - Prob. 17.25CPCh. 17 - Prob. 1MCCh. 17 - Prob. 2MCCh. 17 - Look at the following method header: void...Ch. 17 - Look at the following method header: void...Ch. 17 - Look at the following method header: void...Ch. 17 - Look at the following method header: void...Ch. 17 - Prob. 7MCCh. 17 - Prob. 8MCCh. 17 - Prob. 9MCCh. 17 - The process used by the Java compiler to remove...Ch. 17 - True or False: It is better to discover an error...Ch. 17 - Prob. 12TFCh. 17 - True or False: Type parameters must be single...Ch. 17 - Prob. 14TFCh. 17 - Prob. 15TFCh. 17 - True or False: You cannot create an array of...Ch. 17 - Prob. 17TFCh. 17 - Prob. 18TFCh. 17 - Prob. 1FTECh. 17 - Assume the following is a method header in a...Ch. 17 - public class MyClassT { public static void...Ch. 17 - public class PointT extends Number super Integer {...Ch. 17 - Assume there is a class named Customer. Write a...Ch. 17 - Assume names references an object of the...Ch. 17 - Prob. 3AWCh. 17 - Prob. 4AWCh. 17 - Prob. 5AWCh. 17 - Prob. 6AWCh. 17 - Prob. 7AWCh. 17 - Prob. 1SACh. 17 - Look at the following method header: public T...Ch. 17 - Prob. 3SACh. 17 - Do generic types exist at the bytecode level?Ch. 17 - Prob. 5SACh. 17 - When the compiler encounters a class, interface,...Ch. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardAssignment 2: - Create the first Apex class or program -- In this apex class, we have to create 2 methods -- In the first method, we will take the argument of (account id), and the return type will be (the list of contact). -- In the second method, we will take the argument of (list of account) and the return type will be ( map)arrow_forwardclass displayClass{public:void print();...private:int listLength;int *list;double salary;string name;} Write the definition of the function to overload the assignment operator for the class displayClass.arrow_forward
- Bookstore class uses a dynamic array to hold names book titles. class BookStore { public: BookStore (); BookStore (const Bookstore & b); // POST: object made from b -BookStore (); void insert(string title); // POST: add title to the end of the titles list private: string store; int capacity; int size; }; Describe two different situations in which the copy constructor function is called. Write a short code snippet illustrating each situation. Explain the problem if this class did not provide a deep copy constructor function. // POST: empty object with room for 10 titles // POST: object is destructed // pointer to dynamic array of book titles // capacity of array // number of books used in the bookstorearrow_forwardTrue/ False 1- Class objects can be defined prior to the class declaration. 2- You must use the private access specification for all data members of a class. 3- If an array is partially initialized, the uninitialized elements will be set to zero. 4- A vector object automatically expands in size to accommodate the items stored in it. 5- With pointer variables, you can access but not modify data in other variables. 6- Assuming myValues is an array of int values and index is an int variable, both of the following statements do the same thing. 1. cout header file. 15- To solve a problem recursively, you must identify at least one case in which the problem can be solved without recursion.arrow_forwardProblem 2: Route Planning You are on the development team for a Route Planning application. The team has already developed a class named NavigatorApp for storing and displaying the graph representing the road network. This class has a void displayShortestPath() function which takes the source and destination vertices of the trip and displays the shortest route between them. Using the strategy pattern, draw a class diagram of the system which will allow for the NavigatorApp to display the shortest driving, walking, or cycling route when the displayShortestPath() function is called. You can assume that another developer is in charge of making sure the appropriate strategy is correctly set and for calling the displayShortestPath() function Starter code /* Modify NavigatorApp (if necessary) */ class NavigatorApp { private: Graph map; public: void displayMap(Window * window); void displayShortestPath(Vertex source, Vertex destination, Window * window); /* write pseudo code for this function…arrow_forward
- CONSTRUCTOR// IntSet()// Pre: (none)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements).// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)// Post: True is returned if the invoking IntSet has no relevant// relevant elements, otherwise false is returned.// bool contains(int anInt) const// Pre: (none)// Post: true is returned if the invoking IntSet has anInt as an// element, otherwise false is returned.// bool isSubsetOf(const IntSet& otherIntSet) const// Pre: (none)// Post: True is returned if all elements of the invoking IntSet// are also elements of otherIntSet, otherwise false is// returned.// By definition, true is returned if the invoking IntSet// is empty (i.e., an empty IntSet…arrow_forwardSmart Pointer: Write a smart pointer class. A smart pointer is a data type, usually implemented with templates, that simulates a pointer while also providing automatic garbage collection. It automatically counts the number of references to a SmartPointer<?> object and frees the object of type T when the reference count hits zero.arrow_forwardTrue or False Objects that are instances of a class can be stored in an array.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
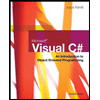
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,