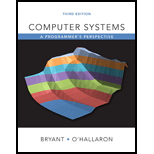
You just started working for a company that is implementing a set of procedures to operate on a data structure where 4 signed bytes are packed into a 32-bit unsigned. Bytes within the word are numbered from 0 (least significant) to 3
(most significant). You have been assigned the task of implementing a function for a machine using two’s-complement arithmetic and arithmetic right shifts with the following prototype:
/* Declaration of data type where 4 bytes are packed
into an unsigned */
typedef unsigned packed_t;
/* Extract byte from word. Return as signed integer */
int xbyte (packed_t word, int bytenum);
That is, the function will extract the designated byte and sign extend it to be a 32-bit int.
Your predecessor (who was fired for incompetence) wrote the following code:
/* Failed attempt at xbyte */
int xbyte (packed_t word, int bytenum)
{
return (word >>(bytenum <<3)) & 0xFF;
}
- A. What is wrong with this code?
- B. Give a correct implementation of the function that uses only left and right shifts, along with one subtraction.

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Additional Engineering Textbook Solutions
Experiencing MIS
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Concepts of Programming Languages (11th Edition)
Introduction to Programming Using Visual Basic (10th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
- Write a C program Test, Debug and execute the C program Write a program that reverses the order of the bits in an unsigned integer value. The program should input the value from the user and call function reverseBits to print the bits in reverse order. Print the value in bits both before and after the bits are reversed to confirm that the bits are reversed properly. Write a program that creates a function multiple that determines if the integer entered from a keyboard is a multiple of some integer x.arrow_forwardPlease use C++ In this lab, you need to write a program which, given a seven digit binary input given below, it will check to see if there is a single bit error using the Hamming Code method discussed in lab. For a sample of Hamming Code, the input: 1110101 will reveal that ‘110’ is the location of the error (meaning the sixth slot in the input), so the corrected number would be 1010101, and the actual message transmitted, would be 1011, which is 11 in decimal. You can use this example to test your program to ensure it’s working correctly. Create a program which can, given a 7-bit long input, written in Hamming Code style, do the three following tasks, displaying the results as output. (1) Determine if there’s an incorrect bit, and if so, where it is. (2) Display what the CORRECT code should look like (if it needs to be corrected.) (3) Display the decimal form of the message that was sent, after any needed correction. Remember, when using Hamming…arrow_forwardIn c++ Now write a program for a double floating pointtype.•What are the number of significant bits that the double’s mantissa can hold, excluding the sign bit.•What is the largest number that a double’s mantissa can hold without roundoff error?•Repeat the program shown on the previous page but set to show where the double’s mantissa starts to exhibit roundoff errors.arrow_forward
- This needs to be done in C Programming. A manufacturing plant has an alarm monitor program that reports any of sixteen possible alarms. A 16-bit variable ALARM is examined, and each 1 bit found corresponds to an active alarm. The alarms are numbered 1 – 16, with the least significant bit (LSB) corresponding to Alarm 1 and the most significant bit (MSB) corresponding to Alarm 16. Prompt for the unsigned short integer ALARM, and then use bitwise logic to determine and display all corresponding active alarms, e.g., output “Alarm 12 Active”.arrow_forwardSolve the below program in C language. Write a program in C to swap two numbers using function. Test Data :Input 1st number : 2Input 2nd number : 4Expected Output : Before swapping: n1 = 2, n2 = 4 After swapping: n1 = 4, n2 = 2arrow_forwardImplement the function (in C or C++) with the following prototype: /** Implement a function which rotates a word left by n-bits, and returns that rotated value. Assume 0 <= n < w Examples: when x = 0x12345678 and w = 32: n=4 -> 0x23456781 n=20 -> 0x67812345 */ unsigned rotate_left(unsigned x, int n); The function should follow the bit-level integer coding rules above. Be careful of the case n = 0.arrow_forward
- Write a MIPS program that will handle calculating minifloat addition for two numbers using the rules discussed in class and storing each of the parts as a binary integer. To do this, each ‘minifloat’ number will need to be represented (I suggest hard coded) as one register for the sign, one for the exponent part, one for the ‘significand’ (which you then convert to the fraction part). Hard coding means here that you don’t need to read in the 6 parts as user input, just have something like li $s0, 0 li $s1, 12 li $s2, 5 which is equivalent to a minifloat of 0 1101 101 = 1.101 * 2^(12-7) = 1.101*2^5 This means your program will be work with at least 6 registers as variables. For testing, show that your program can correctly calculate the Lab 5 X+Y and A+B and then one other example of your choosing. Don’t worry about special cases of 0 0000 000 or 1 1111 111 or similar, but your program should detect overflow. This will involve a lot of (in your head) jumping back and forth between…arrow_forwardthis is COmputer machine architecture ! Help me fix the following code as theres an error. what were trying to accoplish; The assignment is to create a MIPS program that corrects bad data using Hamming codes. The program is to request the user to enter a 12-bit Hamming code and determine if it is correct or not. If correct, it is to display a message to that effect. If incorrect, it is to display a message saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. I will be testing only with single bit errors, so the program should be able to correct my tests just fine. You do not need to worry about multiple bit errors. # This program corrects bad data using Hamming codes # It requests the user to enter a 12-bit Hamming code and determines if it is correct or not # If correct, it displays a message to that effect. If incorrect, it displays a message # saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. # This…arrow_forwardThis in C language Defining a binary number as Program 1, write the function int binToDec(const int bin[]) to convert an eight-bit unsigned binary number to a nonnegative decimal integer. Do not output the decimal integer in the function. Test your function with interactive input. Defining bAnd, bin1, and bin2 as binary numbers as in Program 1 above, write the void function void binaryAnd(int bAnd[], const int bin1[], const int bin2[]) to compute bAnd as the logical AND of the two binary numbers bin1 and bin2. Do not output the binary number in the function. Test your function with interactive input. Program1 in C: #include <stdio.h>int main(){int binNum[8]; // Array to read binary numberlong dec=0,n=0; // variables used to convert binary to decimalint k=0,l=0;long binary=0;int i=1,j=0,remainder=0; //reading the binary number in to the array binNumprintf("Please Enter the first binary number with each bit seperate by at least one space : \n"); scanf("%d %d %d %d %d %d %d…arrow_forward
- please write in ARM UAL and add comment for explanation.. Thank you..; Write a program that swaps 5th~11th bits in data_a with 25th~31th bits in data_b; Your program must work for any data given, not just the example below; In this question, we assmue that the positions of bits count from right to left.; That is, the first bit is the least significant bit.data_a DCD 0x77FFD1D1data_b DCD 0x12345678arrow_forwardUsing C++, write a program that converts an integer to 32-bit two's complement. Add a space between every 8 bits. Do not use external libraries that provide the calculations automatically.Example outputThis program converts an integer to 32-bit two's complement.Enter an integer: 1999Two's complement: 00000000 00000000 00000111 11001111Enter an integer: -65535Two's complement: 11111111 11111111 00000000 00000001arrow_forwardYou are asked to design a 16-bit floating point number system to store the lengths of various man-made objects. This system should work in a similar way as the IEEE754 standard. Assume a value stored in the system denotes the length of an object in centimeters, assume also that the maximum length to be stored is 45845.0 centimeters (i.e. length of the biggest man-made oil-tanker, the “Seawise Giant”). Note: This representation has normalized, de-normalized and special cases as you have seen in IEEE754 standard. Answer the questions below: a) Is sign bit needed in this system? Why yes or why not. b) What is the minimum number of bits needed for the exponent? What is the value of the corresponding bias? Show your steps clearly. If you write the values directly without showing the steps, you will not get any point. c) What is the maximum length the system can represent? Please show your steps clearly, otherwise no point will be given.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
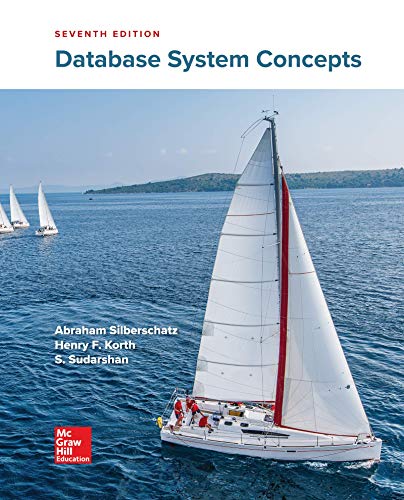
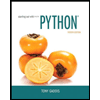
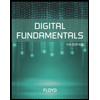
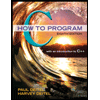
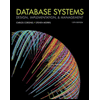
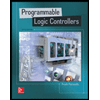