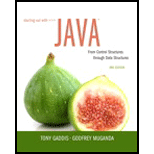
Explanation of Solution
The given program is used to implement stack using linked list.
Logical error:
Logical error is a mistake in a program’s source code that leads to produce an incorrect output. This error is a type of run time error, as it cannot be identified during the compilation of the program. This is a logical mistakes created by the programmer and it is determined when the program produces wrong output.
Error in the given code:
The function “pop()” forgets to remove the value from the stack. That is the statement for removing the element is missing in the function definition. The below given statement should be added in the function definition to produce the correct output.
Correct statement:
/*creates a string variable retValue which holds value of the top element*/
String retValue=top.value;
//assigning the top with the link of next node of the list
top=top.next;
//returning the value at position top in the stack
return retValue;
Corrected code:
/*pop method removes the value from the top position of the stack*/
int pop()
{
/*condition for checking whether the stack is empty*/
...

Want to see the full answer?
Check out a sample textbook solution
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- Book: Java Software Structures Author: John Lewis; Joe Chase Javascript 1. Create a generic type java interface StackADT<T>with the following methods: a.public void push(T element);//Push an element at the top of a stack b.public T pop();//Remove and return an element from the top of a stack c.public T peek();//return the top element without removing it d.public booleanisEmpty();//Return True if a stack is emptye.public int size();//Return the number of elements in a stack f.public String toString();//Print all the elements of a stack 2. Define ArrayStack<T>class which will implementStackADT<T>interface 3. Use your ArrayStack<T>class to compute a postfix expression, for instance, 3 4 * will yield12;3 4 6 + *will yield 30 etc. During computing a postfix expression: a.While you perform a push operation, if the stack is full, generate an exception b.While you perform a pop operation, if the stack is empty, generate an exception c.If two operands are not available…arrow_forward**Use the Stack interface to implement the LinkedStack.java class. Stack.java public interface Stack<E> {/*** Push an element onto the stack.** @param element, a value to be pushed on the stack*/public void push(E element);/*** Pop the top element off the stack.*/public E pop();/*** Return the top element on the stack.*/public E top();/*** Return True if the stack contains no elements.** @return true if there are no elements in the stack*/public boolean isEmpty();} ***In LinkedStack.java, change only The methods with empty bodies Do not use a sentinel node. In this implementation you use an inner class That is, a class that is declared inside of another class. The Node class isn't needed by any other class, so it is declared as a private class inside the LinkedStack class. The Node class has two fields: element and next. Things to note in the class LinkedStack: The EmptyStackExceptionis being used, same as in the ArrayStack. The class StringJoineris imported for use in the…arrow_forward1. Write a generic static method that takes a Stack of any type element as a parameter, pops each element from the stack, and prints it. It should have a type parameter that represents the Stack's element type.arrow_forward
- don't use others answers java 1. Write a generic static method that takes a Stack of any type element as a parameter, pops each element from the stack, and prints it. It should have a type parameter that represents the Stack’s element type.arrow_forwardThe ADT stack lets you peek at its top entry without removing it. For some applications of stacks, you also need to peek at the entry beneath the top entry without removing it. We will call such an operation peek2. If the stack has more than one entry, peek2 returns the second entry from the top without altering the stack. If the stack has fewer than two entries, peek2 throws an exception. Write a linked implementation of a stack class call First_Last_LinkStack.java that includes a method peek2arrow_forwardvoid stack::do(){ for(int i=0li<=topindex/2;i++){ T temp=entry[i]; entry[i]=entry[topindex-i-1]; entry[topindex-1-i]=temp;} } Assume the stack is array based. What is this method do? a. swap the first item with last item b. doesn't do any thing c. replace each item with next item value d. reverse the stackarrow_forward
- Assume an implementation of a STACK that holds integers. Write a method public void CountPosNeg (Stack S) that takes Stack S as a parameter. After the call, the method prints the count of positive integers and negative integers on the stack. Note, the original must not be destroyed. public void CountPosNegarrow_forwardAn ADT linked stack with the top node as its last link is a good example of how this stack might be used. The following methods may be defined without traversing. A (a) pop (b) peekarrow_forwardPlease elaborate on the distinction between the bound and unbounded stack.arrow_forward
- void do(stack s){ stacktemp; while(!s.empty()){ temp.push(s.top(); s.pop();} s=temp;} The stack s is a. destroyed (empty) b. reversed c. not changed d. has rubbish dataarrow_forwardHere is a method for stack operation: function (int a, int b) { if ( (a less than or equal to zero) || (b less than or equal to zero)) return 0; push (b) to stack; return function (a-1, b-1) + stack.pop; } What is the final integer value returned by function call of call (7, 7) ? Must show all the work Show all the recursive calls each time function is called. Must show all the work.arrow_forwardWrite a method of the ArrayBoundedStack class to swap the item at the bottom of the stack with the item at the top of the stack. Do not use any other stack methods in your code. The fields of the ArrayBoundedStack are: protected final int DEFCAP = 100; // default capacity protected T[] stack; // holds stack elements protected int topIndex = -1; // index of top element in stackarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
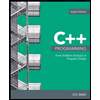