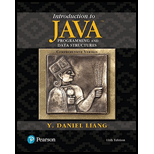
Program to display maximum consecutive increasingly ordered substring
Program Plan:
- Create the class “Exercise22_01”.
- In the main() function,
- Read the input object to read the input from user.
- Call the function maxConsecutivesSortedSubstring()to print the maximum ordered substring.
- In the maxConsecutivesSortedSubstring(),
- Initially assign the maximum length of the substring.
- Use for() loop to execute the whole length of the string.
- Check the position of character at “i” and “i-1” and compare the values.
- If it is lesser, assign the “i” to “current”.
- If the condition not satisfies, then increment the length of maximum consecutive length.
- Use for() loop to iterate the string values.
- Check whether the current length of string is less than the largest string.
- If yes, then assign the current element length to largest subsequence element.
- Construct the character sequence using while() loop at the end of the string.
- Return the string.
- Check the position of character at “i” and “i-1” and compare the values.
- In the maxConsecutivesSortedSubstring1(),
- Use the for loop to iterate the whole length of string.
- Check the position of character at “i” and “i-1” and compare the values.
- Return the sorted substring.
- Use the for loop to iterate the whole length of string.
This program reads the input from user and displays the maximum consecutive increasingly ordered substring.
Program:
//Declare the class Exercise22_01
public class Exercise22_01 {
//Declare the main function
public static void main(String[] args) {
//Create the input object
java.util.Scanner input = new
java.util.Scanner(System.in);
//Read the string
System.out.print("Enter a string: ");
String s = input.nextLine();
/*Call the function maxConsecutiveSortedSubstring() to print the maximum consecutive sorted substring*/
System.out.println("Maximum consecutive substring
is " + maxConsecutiveSortedSubstring(s));
}
/*Define the function maxConsecutiveSortedSubstring()*/
public static String
maxConsecutiveSortedSubstring(String s) {
//Declare the array and assign the length of array
int[] maxConsecutiveLength = new int[s.length()];
//Assign the variable as 0
int current = 0;
//Execute the for loop until the condition fails
for (int i = 1; i < s.length(); i++) {
/*Check whether the character is smaller than
the current character stored in string*/
if (s.charAt(i) <= s.charAt(i - 1)) {
//Assign the “i” value to current variable
current = i;
} else {
/*Execute the for loop until the condition fails*/
for (int j = i - 1; j >= current; j--)
//Increment the sequence of length
maxConsecutiveLength[j]++;
}
}
//Assign the length of sequence at index “i”
int currentMaxLength = maxConsecutiveLength[0];
int index = 0;
//Execute the for loop until the length of string
for (int i = 0; i < s.length(); i++) {
//Check whether the condition is true
if (maxConsecutiveLength[i] > currentMaxLength)
{
/*Assign the maximum sequence length to current length */
currentMaxLength = maxConsecutiveLength[i];
//Assign the index position
index = i;
}
}
//Return the substring
return s.substring(index, index + currentMaxLength + 1);
}
//Define the function for the sorted substring
public static String
maxConsecutiveSortedSubstring1(String s) {
//Assign the current length
int currentMaxLength = 1;
//Assign the last index of sorted substring
int lastIndexOfMaxConsecutiveSortedSubstring = 0;
//Assign the possible length
int possibleMaxLength = 1;
//Execute the for loop until it fails
for (int i = 1; i < s.length(); i++) {
//Check the position of the string
if (s.charAt(i) > s.charAt(i - 1)) {
//Check the condition
if
(lastIndexOfMaxConsecutiveSortedSubstri
ng == i - 1) {
/* Add the max consecutive substring*/
currentMaxLength++;
/*Add the index of max consecutive substring*/
lastIndexOfMaxConsecutiveSortedSub
string++;
//If condition not satisfies
} else {
//Increment the length
possibleMaxLength++;
//Check the condition
if (possibleMaxLength >
currentMaxLength) {
/*Assign the possible length to current maximum length*/
currentMaxLength =
possibleMaxLength;
/*Assign the index of the string*/
lastIndexOfMaxConsecutiveSort
edSubstring = i;
possibleMaxLength = 1;
}
}
}
}
//Return the sorted substring
return
s.substring(lastIndexOfMaxConsecutiveSortedSubstr
ing - currentMaxLength + 1,lastIndexOfMaxConsecutiveSortedSubstring + 1);
}
}
Running time complexity:
The above program executes in
Sample Output:
Enter a string: abcabcdgabmnsxy
Maximum consecutive substring is abmnsxy

Program to display maximum consecutive increasingly ordered substring
Program Plan:
- Create the class “Exercise22_01”.
- In the main() function,
- Read the input object to read the input from user.
- Call the function maxConsecutivesSortedSubstring()to print the maximum ordered substring.
- In the maxConsecutivesSortedSubstring(),
- Initially assign the maximum length of the substring.
- Use for() loop to execute the whole length of the string.
- Check the position of character at “i” and “i-1” and compare the values.
- If it is lesser, assign the “i” to “current”.
- If the condition not satisfies, then increment the length of maximum consecutive length.
- Use for() loop to iterate the string values.
- Check whether the current length of string is less than the largest string.
- If yes, then assign the current element length to largest subsequence element.
- Construct the character sequence using while() loop at the end of the string.
- Return the string.
- Check the position of character at “i” and “i-1” and compare the values.
- In the maxConsecutivesSortedSubstring1(),
- Use the for loop to iterate the whole length of string.
- Check the position of character at “i” and “i-1” and compare the values.
- Return the sorted substring.
- Use the for loop to iterate the whole length of string.
This program reads the input from user and displays the maximum consecutive increasingly ordered substring.
Explanation of Solution
Program:
//Declare the class Exercise22_01
public class Exercise22_01 {
//Declare the main function
public static void main(String[] args) {
//Create the input object
java.util.Scanner input = new
java.util.Scanner(System.in);
//Read the string
System.out.print("Enter a string: ");
String s = input.nextLine();
/*Call the function maxConsecutiveSortedSubstring() to print the maximum consecutive sorted substring*/
System.out.println("Maximum consecutive substring
is " + maxConsecutiveSortedSubstring(s));
}
/*Define the function maxConsecutiveSortedSubstring()*/
public static String
maxConsecutiveSortedSubstring(String s) {
//Declare the array and assign the length of array
int[] maxConsecutiveLength = new int[s.length()];
//Assign the variable as 0
int current = 0;
//Execute the for loop until the condition fails
for (int i = 1; i < s.length(); i++) {
/*Check whether the character is smaller than
the current character stored in string*/
if (s.charAt(i) <= s.charAt(i - 1)) {
//Assign the “i” value to current variable
current = i;
} else {
/*Execute the for loop until the condition fails*/
for (int j = i - 1; j >= current; j--)
//Increment the sequence of length
maxConsecutiveLength[j]++;
}
}
//Assign the length of sequence at index “i”
int currentMaxLength = maxConsecutiveLength[0];
int index = 0;
//Execute the for loop until the length of string
for (int i = 0; i < s.length(); i++) {
//Check whether the condition is true
if (maxConsecutiveLength[i] > currentMaxLength)
{
/*Assign the maximum sequence length to current length */
currentMaxLength = maxConsecutiveLength[i];
//Assign the index position
index = i;
}
}
//Return the substring
return s.substring(index, index + currentMaxLength + 1);
}
//Define the function for the sorted substring
public static String
maxConsecutiveSortedSubstring1(String s) {
//Assign the current length
int currentMaxLength = 1;
//Assign the last index of sorted substring
int lastIndexOfMaxConsecutiveSortedSubstring = 0;
//Assign the possible length
int possibleMaxLength = 1;
//Execute the for loop until it fails
for (int i = 1; i < s.length(); i++) {
//Check the position of the string
if (s.charAt(i) > s.charAt(i - 1)) {
//Check the condition
if
(lastIndexOfMaxConsecutiveSortedSubstri
ng == i - 1) {
/* Add the max consecutive substring*/
currentMaxLength++;
/*Add the index of max consecutive substring*/
lastIndexOfMaxConsecutiveSortedSub
string++;
//If condition not satisfies
} else {
//Increment the length
possibleMaxLength++;
//Check the condition
if (possibleMaxLength >
currentMaxLength) {
/*Assign the possible length to current maximum length*/
currentMaxLength =
possibleMaxLength;
/*Assign the index of the string*/
lastIndexOfMaxConsecutiveSort
edSubstring = i;
possibleMaxLength = 1;
}
}
}
}
//Return the sorted substring
return
s.substring(lastIndexOfMaxConsecutiveSortedSubstr
ing - currentMaxLength + 1,lastIndexOfMaxConsecutiveSortedSubstring + 1);
}
}
Running time complexity:
The above program executes in
is
Enter a string: abcabcdgabmnsxy
Maximum consecutive substring is abmnsxy
Want to see more full solutions like this?
Chapter 22 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Modern Database Management
Starting Out with C++: Early Objects
C++ How to Program (10th Edition)
Artificial Intelligence: A Modern Approach
- Write a public static void function named getLongestName. This function should be designed to take a String [] reference variable and use this to find the longest name in the array. Your function should then return this String.Make a call to this function in your main function passing it namesList at the actual parameter. Use the returned string so that you print out a meaningful message to the screen.arrow_forwardComplete the function that computes the length of a path that starts with the given Point. The Point structure = point on the plane that is a part of a path. The structure includes a pointer to the next point on the path, or nullptr if it's the last point. I'm not sure how to fill in the '?'; especially the while loop I'm not sure how to implement the condition for that.arrow_forwardfunction myCompose(f,g){// TODO: return (f o g);// that is, a function that returns f(g(x)) when invoked on x.}arrow_forward
- In C++ language For number 2 through 4 create an integer array with 100 randomly generated values between 0 and 99; pass this array into all subsequent functions. Place code in your main to call all the methods and demonstrate they work correctly. Using just the at, length, and substr string methods and the + (concatenate) operator, write a function that accepts a string s, a start position p, and a length l, and returns a subset of s with the characters starting at position p for a length of l removed. Don’t forget that strings start at position 0. Thus (“abcdefghijk”, 2, 4) returns “abghijk” Create a function that accepts the integer array described above returns the standard deviation of the values in a. The standard deviation is a statistical measure of the average distance each value in an array is from the mean. To calculate the standard deviation, you first call a second mean function that you need to write (do not use a built in gadget. Then sum the square of the difference…arrow_forwardLinks Bing Remaining Time: 1 hour, 24 minutes, 17 seconds. * Question Completion Status: Р QUESTION 8 bbhosted.cuny.edu/webapps/assessment/take/launch.jsp?course_assessment_id=_2271631_1&course_id=_2 Implement the following: 1) Define a function evenList() with an arbitrary parameter a. P 2) This function accepts any number of integer arguments. 3) evenList() stores all even numbers into a list and returns the list. 4) Call the function with 5, 6, 7, 8, 9, 10 as arguments. 5) Print the result of the function call. Make sure to precisely match the output format below. Write your code in the ANSWER area provided below (must include comments if using code is not covered in the course). Example Output [6, 8, 10] For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). BIUS Paragraph Arial QUESTION 9 Programs s 10pt Click Save and Submit to save and submit. Click Save All Answers to save all answers. く Ev Avarrow_forwardUsing visual studio (C#) create a program, name it PRGYOURNAMEFA1, that implements a search and replace function recursively. Your program should allow a user to enter a string , a substring to be replaced in the entered string and a character/s to replace the found substring Program Structure 1. A main class that implements the logic of the program – name this class TestSearchReplace 2. Add a class named SearchReplace to the main class with two methods, including: a. SearchSubstring()- return method b. ReplaceSubString() - void method The two method should be called using an object in the main class. DONT CREATE THE TWO METHODS IN THE MAIN CLASS Sample Outputarrow_forward
- Instructions: In the code editor, you are provided with a main() function that asks the user for a string and passes this string and the size of this string to a function call of the function, preserveString(). This preserveString() function has the following description: Return type - void Name - preserveString Parameters The string Length of the string Description - this is a recursive function that prints the string repeatedly. Each time it prints the string, it excludes the last character of the string until only one character is left. This preserveString() function has already been partially implemented. Your only task is to add the recursive case of this function. Please Finish the code ASAP: This is my current given code: #include<stdio.h>#include<string.h> #define STR_MAX_SIZE 100 void preserveString(char*, int); int main(void) { char str[STR_MAX_SIZE]; printf("Enter string: "); fgets(str, STR_MAX_SIZE, stdin); preserveString(str,…arrow_forwardIn C++, define a “Conflict” function that accepts an array of Course object pointers. It will return two numbers:- the count of courses with conflicting days. If there is more than one course scheduled in the same day, it is considered a conflict. It will return if there is no conflict.- which day of the week has the most conflict. It will return 0 if there is none. Show how this function is being called and returning proper values. you may want to define a local integer array containing the count for each day of the week with the initial value of 0.Whenever you have a Course object with a specific day, you can increment that count for that corresponding index in the schedule array.arrow_forwardWrite a function to display a pattern as follows: **************** ************** ************... *The function header is void displayPattern(int n)arrow_forward
- Using visual studio (C#) create a program, name it PRGYOURNAMEFA1, that implements a search and replace function recursively. Your program should allow a user to enter a string , a substring to be replaced in the entered string and a character/s to replace the found substring Program Structure1. A main class that implements the logic of the program – name this class TestSearchReplace2. Add a class named SearchReplace to the main class with two methods, including:a. SearchSubstring()- return methodb. ReplaceSubString() - void methodarrow_forwardUsing visual studio (C#) create a program, name it PRGYOURNAMEFA1, that implements a search and replace function recursively. Your program should allow a user to enter a string , a substring to be replaced in the entered string and a character/s to replace the found substring Program Structure1. A main class that implements the logic of the program – name this class TestSearchReplace2. Add a class named SearchReplace to the main class with two methods, including:a. SearchSubstring()- return methodb. ReplaceSubString() - void method The two method should be called using an object in the main class. DONT CREATE THE TWO METHODS IN THE MAIN CLASSarrow_forwardIn java there must be at least two calls to the function with different arguments and the output must clearly show the task being performed. Develop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaks. Develop a function that finds the starting index of the longest subsequence of values that is strictly increasing. For example, given the array {12, 3, 7, 5, 9, 8, 1, 4, 6}, the function…arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
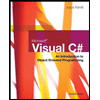