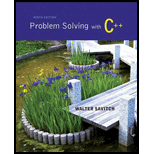
Concept explainers
Listed below are definitions of two classes that use inheritance, code for their implementation, and a main function. Put the code into appropriate files with the necessary include statements and preprocessor statements so that the program compiles and runs. It should output “Circle has radius 2 and area 12.5664”.
class Shape { public: Shape(); Shape(string name); string getName(); void setName(string newName); virtual double getArea() = 0; private: string name; }; Shape::Shape() { name=""; } Shape::Shape(string name) { this->name = name; } string Shape::getName() { return this->name; } void Shape::setName(string newName) { this->name = newName; } class Circle : public Shape { public: Circle(); Circle(int theRadius); void setRadius(int newRadius); double getRadius(); virtual double getArea(); private: int radius; }; Circle::Circle() : Shape("Circle"), radius(0) { } Circle::Circle(int theRadius) : Shape("Circle"), radius(theRadius) { } void Circle::setRadius(int newRadius) { this->radius = newRadius; } double Circle::getRadius() { return radius; } double Circle::getArea() { return 3.14159 * radius * radius; } int main() { Circle c(2); cout << c.getName() << " has radius " << c.getRadius() << " and area " << c.getArea() << endl; return 0; } |
Add another class, Rectangle, that is also derived from the Shape class. Modify the Rectangle class appropriately so it has private width and height variables, a constructor that allows the user to set the width and height, functions to retrieve the width and height, and an appropriately defined getArea function that calculates the area of the rectangle.
The following code added to main should output “Rectangle has width 3 has height 4 and area 12.0”.
Rectangle r(3,4); cout << r.getName() << " has width " << r.getWidth() << " has height " << r.getHeight() << " and area " << r.getArea() << endl; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Concepts Of Programming Languages
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (12th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Software Engineering (10th Edition)
Digital Fundamentals (11th Edition)
- #this is a python program #topic: OOP Design a class called Pokemon using a parameterized constructor so that after executing the following line of code the desired result shown in the output box will be printed. First object along with print has been done for you, you also need to create other objects and print accordingly to get the output correctly. [You are not allowed to change the code below] #Write your code for class here team_pika = Pokemon('pikachu', 'charmander', 90, 60, 10) print('=======Team 1=======') print('Pokemon 1:',team_pika.pokemon1_name, team_pika.pokemon1_power) print('Pokemon 2:',team_pika.pokemon2_name, team_pika.pokemon2_power) pika_combined_power = (team_pika.pokemon1_power + team_pika.pokemon2_power) * team_pika.damage_rate print('Combined Power:', pika_combined_power) #Write your code for subtask 2,3,4 here Output: =======Team 1======= Pokemon 1: pikachu 90 Pokemon 2: charmander 60 Combined Power: 1500 =======Team 2======= Pokemon 1:…arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name in other words. Write a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // Instance created holding 6 parameters which are field with value HealthProfile heartRate = new HealthProfile("James","Kill",1978, 79.5 ,175.5, 2021 ); heartRate.DisplayPatientRecord();// call the patient record method } CLASS HeartProfile------------------- public class HealthProfile { // attibutes which holds the following value private String _FirstName; private String _LastName; private int _BirthYear; private double _Height; private double _Weigth; private int _CurrentYear; public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int…arrow_forwardUser-defined Class: You will design and implement your own data class. The class will store data that has been read as user input from the keyboard (see Getting Input below), and provide necessary operations. As the data stored relates to monetary change, the class should be named MoneyChange. The class requires at least 2 instance variables for the name of a person and the coin change amount to be given to that person. You may also wish to use 6 instance variables to represent amounts for each of the 6 coin denominations (see Client Class below). There should be no need for more than these instance variables. However, if you wish to use more instance variables, you must provide a legitimate justification for their usage in the internal and external documentation.Your class will need to have at least a default constructor, and a constructor with two parameters:one parameter being a name and the other a coin amount. Your class should also provide appropriate get and set methods for…arrow_forward
- Please help with the following: C# .NET change the main class so that the user is the one that as to put the name Write a driver class (app) that prompts for the person’s data input, instantiates an object of class HeartRates and displays the patient’s information from that object by calling the DisplayPatientRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // instance of patient record with each of the 4 parameters taking in a value HeartRates heartRate = new HeartRates("James", "Kill", 1988, 2021); heartRate.DisplayPatientRecord(); // call the method to display The Patient Record } CLASS HeartRATES------------------- class HeartRates { //class attributes private private string _First_Name; private string _Last_Name; private int _Birth_Year; private int _Current_Year; // Constructor which receives private parameters to initialize variables public HeartRates(string First_Name, string Last_Name, int Birth_Year, int Current_Year) { _First_Name =…arrow_forward2019 AP® COMPUTER SCIENCE A FREE-RESPONSE QUESTIONS 2. This question involves the implementation of a fitness tracking system that is represented by the StepTracker class. A StepTracker object is created with a parameter that defines the minimum number of steps that must be taken for a day to be considered active. The StepTracker class provides a constructor and the following methods. addDailySteps, which accumulates information about steps, in readings taken once per day activeDays, which returns the number of active days averageSteps, which returns the average number of steps per day, calculated by dividing the total number of steps taken by the number of days tracked The following table contains a sample code execution sequence and the corresponding results. Statements and Expressions Value Returned Comment (blank if no value) StepTracker tr StepTracker(10000); Days with at least 10,000 steps are considered active. Assume that the parameter is positive. new tr.activeDays () ; No…arrow_forwardConvert the pseudocode into Python. 1. Pet ClassDesign a class named Pet, which should have the following fields:■ name: The name field holds the name of a pet.■ type: The type field holds the type of animal that a pet is. Example values are "Dog", "Cat", and "Bird".■ age: The age field holds the pet’s age.2. The Pet class should also have the following methods:■ setName: The setName method stores a value in the name field.■ setType: The setType method stores a value in the type field.■ setAge: The setAge method stores a value in the age field.■ getName: The getName method returns the value of the name field.■ getType: The getType method returns the value of the type field.■ getAge: The getAge method returns the value of the age field.3. Once you have designed the class, design a program that creates an object of the class and prompts the user to enter the name, type, and age of his or her pet. This data should be stored in the object. Use the…arrow_forward
- Classes - Continued... Write a definition of a class and write a program that implements all the functionality of the class that has the following properties. The Main Program should invoke all functionality of this class, i.e., secretType. Input: from a file - the person's name and age and weight and height Output: The Original input and based on their BMI, their weight status. The name of the class is secretType. a. The class secretType has four member variables: name (a string), age and weight (int), height (double) b. The class secretType has the following member functions: (Make the accessor functions const) с. i. print – outputs the data in the member variables in a nicely formatted ii. setName - sets the name iii. setAge – sets the age iv. setWeight – sets the weight setHeight – sets the height V. vi. getName – value returning function returns the name vii. getAge – value returning function returns the age viii. getHeight – value returning function returns the height ix.…arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // instance of patient record with each of the 4 parameters taking in a value HeartRates heartRate = new HeartRates("James", "Kill", 1988, 2021); heartRate.DisplayPatientRecord(); // call the method to display The Patient Record } CLASS HeartRATES------------------- class HeartRates { //class attributes private private string _First_Name; private string _Last_Name; private int _Birth_Year; private int _Current_Year; // Constructor which receives private parameters to initialize variables public HeartRates(string First_Name, string Last_Name, int Birth_Year, int Current_Year) { _First_Name = First_Name;…arrow_forwardThe class definition for an Item is given in the image UML diagram below Note: getInfo() returns a string containing ALL of the state information neatly organized. Part 1: Create a class based on the specification above. Reminder: – means private and + means public. Part 2: a)Write a function/method called “addItem” that takes: An array of items The number of items in the array An integer representing a barcode A string representing the name of an item A string representing the description of an item A double value representing the price The function must create and add the item to the array if there is space. The function must return “true” if the addition was successful and “false” otherwise. b)Write a function/method called “listItems” that takes: An array of items The number of items in the array The function must return a string containing the information on each item in the array. c)Write a function called “pricelookup” that takes : An array of items The number of…arrow_forward
- User-defined Class:You will design and implement your own data class. The class will store data that has been read asuser input from the keyboard (see Getting Input below), and provide necessary operations. As thedata stored relates to monetary change, the class should be named Change. The class requires atleast 2 instance variables for the name of a person and the coin change amount to be given to thatperson. You may also wish to use 4 instance variables to represent amounts for each of the 4 coindenominations (see Client Class below). There should be no need for more than these instancevariables. However, if you wish to use more instance variables, you must provide legitimatejustification for their usage in the internal and external documentation.Your class will need to have at least a default constructor, and a constructor with two parameters:one parameter being a name and the other a coin amount. Your class should also provide appropriateget and set methods for client usage. Other…arrow_forwardbuild a student class implement the student class with the following instance variables: * id * firstName *lastName *dateOfBirth * Major create an __init__ function adn initialize all the fields make the "major" an option field and set a default value to "undefined" create a setter and getter function for all these five variables create another function: "print_student_info()" which prints 5 pieces of information: id, first name, last name, date of birth, and major. Make sure to have proper formatting done for printing these 5 things. Use the student class Task 1: create an empty list named "all_students" Task 2: create a variable named "id" and initialize it to 100 (some default value to start with, next id would be 101) Task 3: ask the user for input "How many students:" Task 4: Now run a for loop based on that input number and do the following things for each iteration: - get input of student's first name, last name, date of birth, and major - user should be able to skip the…arrow_forwardPython Programming2. Write a class named Pet, which should have the following data attributes:(a). __name (for the name of a pet)__animal_type (for the type of animal that a pet is. Example values are ‘Dog’, ‘Cat’, and ‘Bird’)__age (for the pet’s age)The Pet class should have an __init__ method that creates these attributes. It should also have the following methods:(b). set_name: This method assigns a value to the __name field.set_animal_type: This method assigns a value to the __animal_type field.set_age: This method assigns a value to the __age field.get_name: This method returns the value of the __name field.get_animal_type: This method returns the value of the __animal_type field.get_age: This method returns the value of the __age field.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
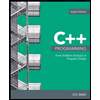