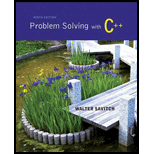
Banks have many different types of accounts, often with different rules for fees associated with transactions such as withdrawals. Customers are allowed to transfer funds between accounts incurring the appropriate fees associated with withdrawal of funds from one account.
Write a
For the classes, create a base class called BankAccount that has the name of the owner of the account (a string) and the balance in the account (double) as data members. Include member functions deposit and withdraw (each with a double for the amount as an argument) and accessor functions getName and getBalance. Deposit will add the amount to the balance (assuming the amount is nonnegative) and withdraw will subtract the amount from the balance (assuming the amount is nonnegative and less than or equal to the balance). Also create a class called MoneyMarketAccount that is derived from BankAccount. In a MoneyMarketAccount the user gets two free withdrawals in a given period of time (don’t worry about the time for this problem). After the free withdrawals have been used, a withdrawal fee of $1.50 is deducted from the balance per withdrawal. Hence, the class must have a data member to keep track of the number of withdrawals. It also must override the withdraw definition. Finally, create a CDAccount class (to model a Certificate of Deposit) derived from BankAccount that in addition to having the name and balance also has an interest rate. CDs incur penalties for early withdrawal of funds. Assume that a withdrawal of funds (any amount) incurs a penalty of 25% of the annual interest earned on the account. Assume the amount withdrawn plus the penalty are deducted from the account balance. Again, the withdraw function must override the one in the base class. For all three classes, the withdraw function should return an integer indicating the status (either ok or insufficient funds for the withdrawal to take place). For the purposes of this exercise, do not worry about other functions and properties of these accounts (such as when and how interest is paid).

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out With Visual Basic (8th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- Banks have many different types of accounts, often with different rules for fees associated with transactions such as withdrawals. Customers are allowed to transfer funds between accounts incurring the appropriate fees associated with withdrawal of funds from one account. Write a program with a base class for a bank account and two derived classes (as described below) representing accounts with different rules for withdrawing funds. Also write a function that transfers funds from one account (of any type) to another. A transfer is a withdrawal from one account and a deposit into the other. Since the transfer can be done at any time with any type of account, the withdraw function in the classes must be virtual. Write a main program that creates three accounts (one from each class) and tests the transfer function. For the classes, create a base class called BankAccount that has the name of the owner of the account (a string) and the balance in the account (double) as data members.…arrow_forwardObject oriented programming C++ Use Classes Write a C++ program in which each flight is required to have a date of departure, a time of departure, a date of arrival and a time of arrival. Furthermore, each flight has a unique flight ID and the information whether the flight is direct or not.Create a C++ class to model a flight. (Note: Make separate classes for Date and Time and use composition).add two integer data members to the Flight class, one to store the total number of seats in the flight and the other to store the number of seats that have been reserved. Provide all standard functions in each of the Date, Time and Flight classes (Constructors, set/get methods and display methods etc.).Add a member function delayFlight(int) in the Flight class to delay the flight by the number of minutes pass as input to this function. (For simplicity, assume that delaying a flight will not change the Date). Add a member function reserveSeat(int) which reserves the number of seats passed as…arrow_forwardprogramming language :c++ make a program in c++ to store hiring date and date of birth for Managers and Employees. You need to create a Date class for this purpose. Create objects of Date class in Manager and Employee classes to store respective dates. You need to write print function in Manager and Employee classes as well to print all information of Managers and Employees. You need to perform composition to implement this task. Create objects of Manager and Employee classes in main function and call print function for both objects. Print Date class here. Print updated Manager class here. Print updated Employee class here. Print main function here.arrow_forward
- PYTHON PROGRAMMING Write a student class that will keep track of the grades of students and generates a final mark. You should store three quiz marks, each out of a total of 20 marks, one midterm score out of a total of 50 marks, one final project out of 100 and an overall score with a letter grade associated with it. Further, create the respected accessor and mutator methods necessary. The function calculateOverallScore() should calculate the weight as follows; quizzes are 15% total, midterm is 35% total and final is worth 50% for a total of 100% when summed up. The function finalLetterGrade() should return the letter based off the following: 100-90 è A, 90-80 è B, 70-80 è C, 60-70 è D, 0 – 60 è F When complete create a student object, set the marks for quizzes/midterm/final and print the letter and final grade to the console.arrow_forwardCreate a class Person with data member Name, age, address and citizenship number. Write a constructor to initialize the value of a person. Assign citizenship number if the age of the person is greater than 16 otherwise assign value zero to citizenship number. Also create a function to display the values.arrow_forwardUsing C++ Write a C++ program which uses a class that contains the following members: the name of a student, one midterm score, one final score, and one lab project score. The class also contains a member function that finds the average of the three scores and displays a grade based on that average (A for 90 and above, B for 80 and above, C for 70 and above, D for 60 and above, and F for anything less than 60). The class also contains a member function that displays a pass if the grade is D or above. Your program using overloading prints the grades of students, and names of the students.arrow_forward
- Create a class Person with data members Name, Age and Address. Create another class Teacher with data members Qualification and Department. Also create another class Student with data member Program and Semester. Both classes are inherited from the class Person. Every class has at least one constructor which uses base class constructor. Create member function Show Data () in each to display the information of the class. C++arrow_forwardThere should be a file for each. One file for EACH class(2 files), their function files (2 files) and a main file(1 file). In total, 5 files and its a class not a struct.arrow_forwardC++ Personal Information Class: Design a class that holds the following personal data: name, age, and phone number. Write appropriate accessor and mutator functions. Demonstrate the class by writing a program that creates three instances of it. One instance should hold your information, and the other two should hold your friend's or family member's information.arrow_forward
- Create a class: Doctor, with private data members: id, name, specialization and salary with public member function: get_data () to take input for all data members, show_data () to display the values of all data members and get specialization () to return specialization. Write a program to write for detail of n doctors by allocating the memory at run time only. Write the program in c++ language.arrow_forwardC++ Create a simple employee class that supports two classifications of employees, salaried and hourly. All employees have four data members: name, employee number, birth date, and date hired. Salaried employees have a monthly salary and an annual bonus, which is stated as a percentage in the range of 0 to 10 percent. Hourly employees have an hourly wage and an overtime rate which ranges from 50 to 100 percent.arrow_forward1. Declare a class student with id, name and percentage 2. Make a public function average in class student which calculates the average student marks in 5 courses using arrays. 3. Define the object of class in main() 4. Get the values of studentlD, name and percentage from the user 5. Access method using class object and display average. 6. Print the values obtained from the user 7. Stop the program.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
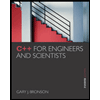
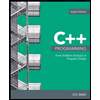